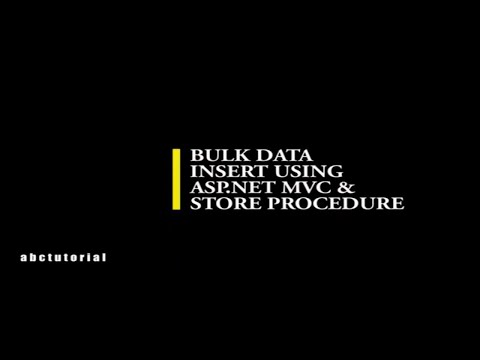
Step-1:
- Add a method inside HomeController.cs called Index
public ActionResult Index()
{
return View();
}
- This code will be add in Index.cshtml Put the html code for design your page using bootstrap
@{
ViewBag.Title = "Home Page";
}
<br />
<br />
<div class="row">
<form>
<div class="form-group row">
<label class="col-sm-2 col-form-label">Employee ID</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="emp_id" placeholder="Enter employee id">
</div>
</div>
<div class="form-group row">
<label class="col-sm-2 col-form-label">Employee name</label>
<div class="col-sm-10">
<input type="text" class="form-control" id="emp_name" placeholder="Employee name">
</div>
</div>
<div class="form-group row">
<label class="col-sm-2 col-form-label">Employee bonus amount</label>
<div class="col-sm-10">
<input type="number" class="form-control" id="emp_bonus" placeholder="Employee bonus amount">
</div>
</div>
<a href="#" id="addNewBtn" class="btn btn-primary col-lg-offset-2"><i class="glyphicon glyphicon-plus-sign"></i> Add New</a>
</form>
<br />
<table class="table table-bordered">
<thead>
<tr>
<th scope="col">Employee ID</th>
<th scope="col">Employee Name</th>
<th scope="col">Employee Bonus Amount</th>
</tr>
</thead>
<tbody id="tbl_list">
</tbody>
</table>
<a href="#" id="autoGenerateBtn" class="btn btn-success col-lg-offset-2"><i class="glyphicon glyphicon-check"></i> Start Auto Generation</a>
</div>
- This JS code will be add in Index.cshtml Put the html code for design your page using bootstrap
<script>
$(document).ready(function () {
var arrayOfEmp = [];
$(document).on("click", "#addNewBtn", function () {
var empObj = {
EmployeeId: $("#emp_id").val(),
EmployeeName: $("#emp_name").val(),
EmployeeBonusAmount: $("#emp_bonus").val()
};
arrayOfEmp.push(empObj);
$("#tbl_list").append('<tr><td>' + empObj.EmployeeId + '</td><td>' + empObj.EmployeeName + '</td><td>' + empObj.EmployeeBonusAmount + '</td></tr>');
});
$(document).on("click", "#autoGenerateBtn", function () {
$.ajax({
type: "POST",
url: "/Home/SaveData",
data: JSON.stringify(arrayOfEmp),
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (r) {
alert(r + " record(s) inserted.");
}
});
});
});
</script>
Step-2:
- Create a class inside the Model folder EmployeeBonusDetail.cs
public class EmployeeBonusDetail
{
public string EmployeeId { get; set; }
public string EmployeeName { get; set; }
public decimal EmployeeBonusAmount { get; set; }
}
- Add a method SaveData to save your data passing from JS.
public JsonResult SaveData(List<EmployeeBonusDetail> listOfDataToSave)
{
List<string> savedRecord = new List<string>();
try
{
DataTable tvp = new DataTable();
tvp.Columns.Add(new DataColumn("Emp_Id", typeof(string)));
tvp.Columns.Add(new DataColumn("Emp_Name", typeof(string)));
tvp.Columns.Add(new DataColumn("Emp_Bonus", typeof(string)));
// populate DataTable from my List here
foreach (var item in listOfDataToSave)
tvp.Rows.Add(new object[] { item.EmployeeId, item.EmployeeName, item.EmployeeBonusAmount });
SqlConnection conn = new SqlConnection("Data Source=.;Initial Catalog=TechnicalTest;Integrated Security=True");
SqlCommand cmd = new SqlCommand("dbo.SaveEmpBonusRecord", conn);
cmd.CommandType = CommandType.StoredProcedure;
SqlParameter tvparam = cmd.Parameters.AddWithValue("@arrayList", tvp);
tvparam.SqlDbType = SqlDbType.Structured;
tvparam.Direction = ParameterDirection.Input;
tvparam.TypeName = "dbo.tpEmpBonus_List";
conn.Open();
SqlDataReader myReader = cmd.ExecuteReader();
while (myReader.Read())
{
savedRecord.Add(myReader.GetString(0));
}
myReader.Close();
}
catch (Exception ex)
{
savedRecord.Add(ex.Message);
}
return Json(savedRecord, JsonRequestBehavior.AllowGet);
}
Step-3:
- Create a User defined table type called tpEmpBonus_List
CREATE TYPE [dbo].[tpEmpBonus_List] AS TABLE(
[Emp_Id] [varchar](200) NULL,
[Emp_Name] [varchar](200) NULL,
[Emp_Bonus] [decimal](20, 2) NULL
)
- Create Storeprocedure called SaveEmpBonusRecord.
GO
ALTER PROCEDURE [dbo].[SaveEmpBonusRecord]
@arrayList AS dbo.tpEmpBonus_List READONLY
AS
BEGIN
--This is for inserting bulk records
INSERT INTO [Employee].[EmployeeBonusDetail]
([EmployeeId]
,[EmployeeName]
,[EmployeeBonusAmount])
SELECT
Emp_Id as EmployeeId, Emp_Name as EmployeeName, Emp_Bonus as EmployeeBonusAmount
FROM
@arrayList
--This is for return saved records
SELECT
Emp_Name
FROM
@arrayList
END