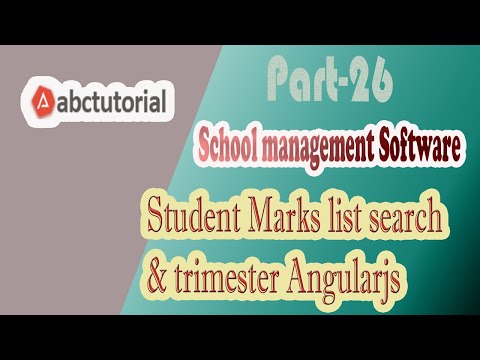
In this post, I would like to Part 26 Student Marks list show by search student name and trimester Jquery & Angular js in ASP.NET MVC.
Step-1: In StudentMarksList Page with writing the below code.
Go to Solution Explorer > Views Folder > StudentMark Folder > open StudentMarksList Page.
<div class="row">
<div class="col-md-4">
<div class="form-group">
<label>Student ID & Name</label>
<select class="form-control" ng-model="StudentCourseOfferDAO.StudentId" id="StudentId">
<option ng-repeat="e in StudentIDNO" value="{{e.StudentId}}">{{e.StudentIdNO}}</option>
</select>
</div>
</div>
<div class="col-md-4">
<div class="form-group">
<label>Trimester Name</label>
<select class="form-control" ng-model="StudentCourseOfferDAO.TrimesterInfoId" id="TrimesterInfoId">
<option ng-repeat="e in Trimester" value="{{e.TrimesterInfoId}}">{{e.TrimesterInfoName}}</option>
</select>
</div>
</div>
<div class="col-md-4">
<div class="form-group">
<br />
<button type="button" class="btn btn-success" onclick="GetList()">Search</button>
</div>
</div>
</div>
Step-2: Search Button click to load Student Marks List in table like as below.
<div class="row">
<div class="col-md-12">
<table class="table table-responsive" id="dtTable">
<thead>
<tr>
<td>SL#</td>
<td>Course Name</td>
<td>Marks</td>
<td>Out of Marks</td>
</tr>
</thead>
<tbody id="dtTableBody">
</tbody>
</table>
</div>
</div>
Step-3: Search Button click to load Student Marks List for below scripts GetList() function.
<script src="~/Scripts/angular.min.js"></script>
<script src="~/Scripts/AngularController/StudentCourseOfferJS.js"></script>
<script>
function GetList() {
var prm = "";
if ($('#StudentId').val() != "") {
prm = prm + " and StudentId ='" + $('#StudentId').val() + "'";
}
if ($('#TrimesterInfoId').val() != "") {
prm = prm + " and TrimesterInfoId ='" + $('#TrimesterInfoId').val() + "'";
}
var urlPath = '@Url.Action("GetStudentMarksList", "StudentMark")';
$.ajax({
url: urlPath,
dataType: 'json',
data: { prm: prm},
type: "Get",
async: true,
success: function (data) {
var result = JSON.parse(data);
var row = "";
var tMark = 0;
var tMarksOutOf = 0;
$('#dtTableBody').html("");
for (var i = 0; i < result.length;i++) {
row +="<tr>";
row += "<td>" + (i + 1) + "</td>";
row += '<td> ' + result[i].CourseName+ '</td>';
row += "<td> " + result[i].Marks + "</td>";
row += "<td>" + result[i].MarksOutOf + "</td>";
tMark = parseFloat(tMark) + parseFloat(result[i].Marks);
tMarksOutOf = parseFloat(tMarksOutOf) + parseFloat(result[i].MarksOutOf);
row += "</tr>";
}
row += "<tr>";
row += '<td> ' + '</td>';
row += '<td> Total:' + '</td>';
row += "<td > " + tMark + "</td>";
row += "<td > " + tMarksOutOf + "</td>";
row += "</tr>";
$('#dtTableBody').html(row);
},
error: function (data) {
alert("Operation Faild!!");
}
})
}
</script>
Step-4: StudentMarkController Add GetStudentMarksList() ActionResult for Load Data. Go to Solution Explorer > Controllers Folder > StudentMarkController.css controller with writing the below code.
public ActionResult GetStudentMarksList(string prm)
{
DataTable dr = aDal.GetStudentMarksListDAL(prm);
string jJSONresult;
jJSONresult = JsonConvert.SerializeObject(dr);
return Json(jJSONresult, JsonRequestBehavior.AllowGet);
}
Step-5: in StudentMarksEntryDAL add GetStudentMarksListDAL() for Load Data. Go to Solution Explorer > DAL Folder > create StudentMarksEntryDAL.css with writing the below code.
public DataTable GetStudentMarksListDAL(string prm)
{
SqlCommand com = new SqlCommand("sp_StudentMarksListLoadByParm", conn);
com.CommandType = CommandType.StoredProcedure;
com.Parameters.AddWithValue("@prm", prm);
SqlDataAdapter da = new SqlDataAdapter(com);
DataTable dss = new DataTable();
da.Fill(dss);
return dss;
}
Step-6: Create store Procedure for Load Student Marks List.
Go to SQL Server 2014 > dbStudentMangeSystem database> Programmability> stored procedures> Select New> stored procedure>Create sp_StudentCourseOfferListLoadByParm
with writing the below code.
create proc [dbo].[sp_StudentMarksListLoadByParm]
@prm nvarchar(max)
as
begin
Declare @Query nvarchar(max)
set @Query ='select co.CourseName, * from tblStudentMarksEntry
left join tblCourse co on tblStudentMarksEntry.CourseId=co.CourseId
where StudentMarksEntryId is not null '+@prm
end
exec(@Query)
Step-7: Run Application.