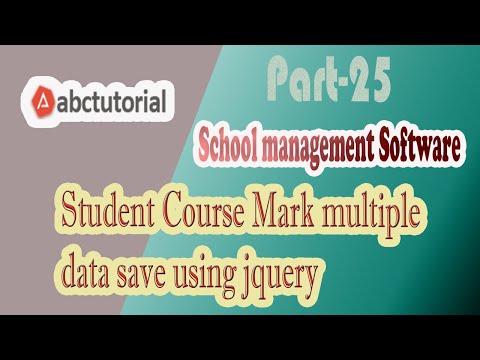
In this post, I will show Student Course Mark multiple data save using jquery with Stored Procedure & Angular js in ASP.NET MVC.
Step-1: In StudentMarksEntry Page with writing the below script for Save Data.
For save data we need a save() function with writing the below script
function Save() {
var jsonData = {};
jsonData["FakeId"] = '1';
//jsDt["TrimesterInfoId"] = $('#TrimesterInfoId').val();
var jsonObjs = [];
$('#dtTable tbody tr').each(function (id) {
var theobj = {};
id++;
var CorsId = $("input[name='CourseList[" + id + "].hfCourseId']").val();
var Mark = $("input[name='MarkList[" + id + "].txtMark']").val();
var OutMark = $("input[name='OutMarkList[" + id + "].txtOutMark']").val();
theobj["CourseId"] = CorsId;
theobj["Marks"] = Mark;
theobj["MarksOutOf"] = OutMark;
theobj["StudentId"] = $('#StudentId').val();
theobj["TrimesterInfoId"] = $('#TrimesterInfoId').val();
jsonObjs.push(theobj);
});
jsonData["StudentMarksEntryDAOLists"] = jsonObjs;
var urlPath = '@Url.Action("Save_Info", "StudentMark")';
$.ajax({
data: JSON.stringify(jsonData),
url: urlPath,
type: "POST",
contentType: "application/json; charset=utf-8",
dataType: "json",
success: function (result) {
alert("Operation Successfully Done")
var url = '@Url.Action("StudentMarksEntry", "StudentMark")'
window.location.href = url;
},
error: function (data) {
alert("Operation Faild!!");
}
})
}
Step-2: In StudentMarkController for insert Student marks add Save_Info().
Go to Solution Explorer > Controllers Folder > create StudentMarkController.css controller with writing the below code.
public ActionResult Save_Info(StudentMarksEntryDAO ADao)
{
string Mes = "";
try
{
aDal.AddNewInfoDAL(ADao);
Mes = "Operation Success!!";
}
catch (Exception ex)
{
Mes = "Error";
}
return Json(Mes, JsonRequestBehavior.AllowGet);
}
Step-3: Create StudentMarksEntryDAO & StudentMarksEntryDAOList Data object.
Go to Solution Explorer > Controllers Folder > StudentMarkController.css controller with writing the below code.
public class StudentMarksEntryDAO
{
public int FakeId { get; set; }
public List<StudentMarksEntryDAOList> StudentMarksEntryDAOLists { get; set; }
}
public class StudentMarksEntryDAOList
{
public int StudentMarksEntryId { get; set; }
public int CourseId { get; set; }
public int TrimesterInfoId { get; set; }
public int StudentId { get; set; }
public decimal Marks { get; set; }
public decimal MarksOutOf { get; set; }
}
Step-4: Create StudentMarksEntryDAL for add AddNewInfoDAL().
Go to Solution Explorer > DAL Folder > create StudentMarksEntryDAL.css with writing the below code.
public void AddNewInfoDAL(StudentMarksEntryDAO aDao)
{
foreach(var item in aDao.StudentMarksEntryDAOLists)
{
SqlCommand com = new SqlCommand("sp_Insert_StudentMarksEntry_Info", conn);
com.CommandType = CommandType.StoredProcedure;
com.Parameters.AddWithValue("@StudentId", item.StudentId);
com.Parameters.AddWithValue("@TrimesterInfoId", item.TrimesterInfoId);
com.Parameters.AddWithValue("@CourseId", item.CourseId);
com.Parameters.AddWithValue("@Marks", item.Marks);
com.Parameters.AddWithValue("@MarksOutOf", item.MarksOutOf);
conn.Open();
com.ExecuteNonQuery();
conn.Close();
}
}
Step-5: Create store Procedure for Save Student M arks Entry.
Go to SQL Server 2014 > dbStudentMangeSystem database> Programmability> stored procedures> Select New> stored procedure>Create sp_StudentCourseOfferListLoadByParm with writing the below code.
create PROCEDURE [dbo].[sp_Insert_StudentMarksEntry_Info]
-- Add the parameters for the stored procedure here
@CourseId int=null ,
@TrimesterInfoId int=null ,
@StudentId int=null ,
@Marks decimal=null ,
@MarksOutOf decimal=null
AS
BEGIN
INSERT INTO [dbo].[tblStudentMarksEntry]
([CourseId]
,[TrimesterInfoId]
,[StudentId]
,[Marks]
,[MarksOutOf])
VALUES
(@CourseId
,@TrimesterInfoId
,@StudentId
,@Marks
,@MarksOutOf)
END
Step-4: Run Application.