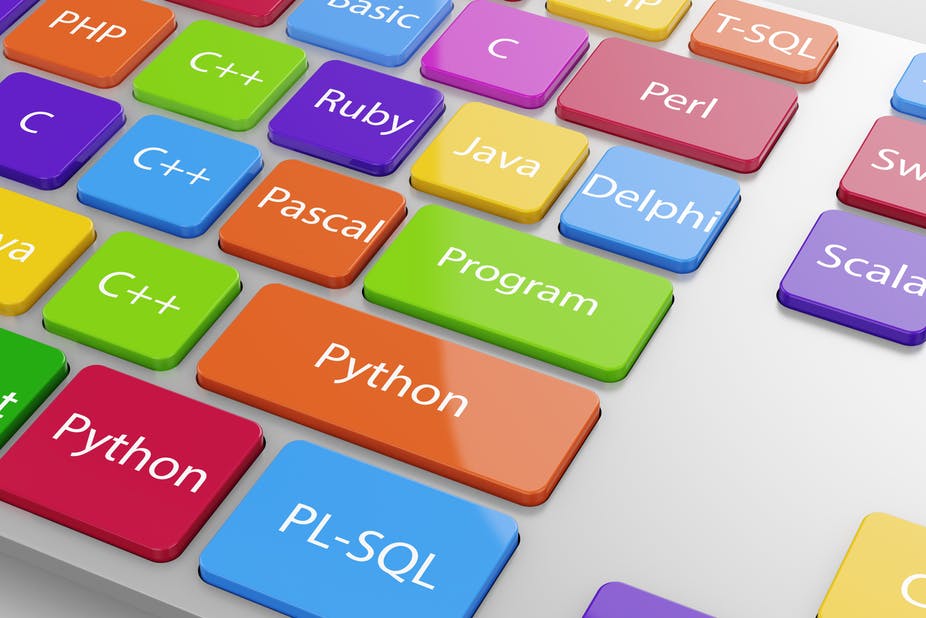
A Method is nothing but block of code. Some time it’s called function. When the function present under the Class it’s called Method. Method Execute by the Calling process. How to identify which is Method? Generally, Method has ‘()’ and ‘{}’ called method body. When we write the Method two things are Understand:
- Method Define
- Method Calling
Discuss the two things by the real-life example:
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Reflection;
using testForClass1;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
namespace testFor
{
public class Students
{
//define the method but not call the method
public void GetStd()
{
Console.WriteLine("Student one print !");
}
static void Main(string[] args)
{
Console.Read();
}
}
}
There is a two Method one is GetStd and another is Main.
Main Method responsible for execute the code Line by Line. So Main Method doesn’t execute the GetStd() Method, because this GetStd() not Call into Main() Method. It is just Define.
Now Method Calling:
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Reflection;
using testForClass1;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
namespace testFor
{
public class Students
{
//define the method but and call the method
public void GetStd()
{
Console.WriteLine("Student one print !");
}
static void Main(string[] args)
{
// call the method using this code
Students obj = new Students();
obj.GetStd();
Console.Read();
}
}
}
First create object of Students class than call the GetStd() Method. GetStd() method responsible to Print this context "Student One Print !" .We can see the Output on console window.