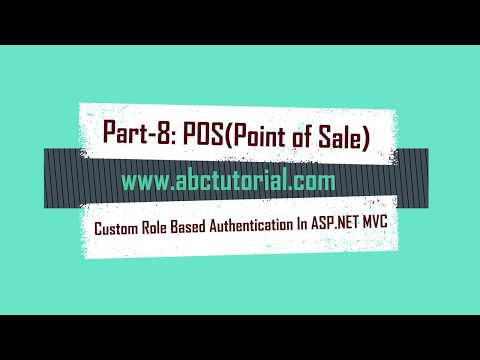
Part-1: Point of Sale(POS) Inventory Super Shop Management System using ASP.NET MVC
Part-2: Point of Sale(POS) Setup template to your project | Super Shop Management System|ASP.NET MVC
Part-3: Point of Sale(POS) Database & table analysis | Super Shop Management System|ASP.NET MVC
Part-4: Point of Sale(POS) Database Create in SQL Server | Super Shop Management System|ASP.NET MVC
Part-5: Point of Sale(POS) Login using AJAX ASP.NET MVC | JQUERY
Part-6: Point of Sale(POS) Login and Authorization with Session variables in ASP.NET | JQUERY | AJAX
Part-7: Point of Sale(POS) Convert Password to MD5 Hash string in ASP.NET | Encrypt password in ASP.NET MVC C#
Part-8: Point of Sale(POS) Role based authentication and authorization in ASP.NET MVC|Redirect to not found page if not authorized|C#
Part-9: Point of Sale(POS) Create user & Account management UI UX in ASP.NET MVC
Part-10: Point of Sale(POS) User Creation & user registration using ASP.NET MVC | Jquery | Ajax
Part-11: Point of Sale(POS) Get user list using ASP.NET MVC | Jquery | Ajax
Part-12: Point of Sale(POS) Update user using ASP.NET MVC | Jquery | Ajax
Part-13: Inventory and POS Login logout session using ASP.NET | Supershop management system
Part-14: Inventory Category CRUD Create,Retrieve,Update,View | POS Category CRUD using ASP.NET JQuery AJAX
Part-15: Inventory and POS batch tracking and control table create in SQL Server 2012
Part-16: Inventory Product CRUD List | Point of sale Products Crud using asp.net MVC
Part-17: Inventory and POS Batch CRUD using asp.net MVC JQUERY AJAX | CSharp
Part-18: Inventory management and stock control using asp.net mvc | Jquery AngularJs
Part-19: Inventory & POS Stock edit and validation using asp.net AngularJS
POS 20: Inventory & POS AngularJS error fix
POS-21: Inventory & POS Invoice template setup using Bootstrap
POS-22: Invoice Adding input fields & adding rows Dynamically using Javascript | ASP.NET MVC | AngularJs
POS-23: Inventory Onclick get selected data & Calculate line total using Javascript ASP.NET MVC JQUERY
POS-24: Inventory Invoice Configuration and Calculation using JavaScript AngularJS ASP.NET MVC
POS-25: Inventory sale from invoice using ASP.NET MVC | Jquery AngularJS
POS-26: Get data using ASP.NET MVC AngularJS JQUERY
POS-27: Invoice sales page edit using ASP.NET MVC JQUERY AngularJS
POS-28: Invoice vat calculation discount calculation using Javascript ASP.NET MVC | Invoice crud asp.net
POS-29: Invoice calculate subtotal add row remove row using AngularJS ASP.NET MVC
See previous article/video before starting this
Step-1:
- Create a table name “RolePermission”
- Table property will be like below
Id - int – Primary key with auto incremented
Role - String
Tag – String
- Table create script is given below
CREATE TABLE [dbo].[RolePermission](
[Id] [int] IDENTITY(1,1) NOT NULL,
[Role] [nvarchar](50) NULL,
[Tag] [nvarchar](50) NULL,
CONSTRAINT [PK_RolePermission] PRIMARY KEY CLUSTERED
(
[Id] ASC
)WITH (PAD_INDEX = OFF, STATISTICS_NORECOMPUTE = OFF, IGNORE_DUP_KEY = OFF, ALLOW_ROW_LOCKS = ON, ALLOW_PAGE_LOCKS = ON) ON [PRIMARY]
) ON [PRIMARY]
- Update new added table to ADO.NET Entity data model by refreshing [for more see the video]
Step-2:
- Add a new Action-Method named “AccessDenied” inside the Home controller.
public ActionResult AccessDenied()
{
return View();
}
- Add a view named “AccessDenied.cshtml” under “Home” folder. Paste this below code
@{
ViewBag.Title = "AccessDenied";
}
<h2>You are not authorized!</h2>
- Replace below code to AuthorizationFilter class
public void OnAuthorization(AuthorizationContext filterContext)
{
POS_TutorialEntities db = new POS_TutorialEntities();
string username = Convert.ToString(System.Web.HttpContext.Current.Session["Username"]);
string role = Convert.ToString(System.Web.HttpContext.Current.Session["Role"]);
string actionName = filterContext.ActionDescriptor.ActionName;
string controllerName = filterContext.ActionDescriptor.ControllerDescriptor.ControllerName;
string tag = controllerName + actionName;
if (filterContext.ActionDescriptor.IsDefined(typeof(AllowAnonymousAttribute), true)
|| filterContext.ActionDescriptor.ControllerDescriptor.IsDefined(typeof(AllowAnonymousAttribute), true))
{
// Don't check for authorization as AllowAnonymous filter is applied to the action or controller
return;
}
// Check for authorization
if (System.Web.HttpContext.Current.Session["Username"] == null)
{
filterContext.Result = new HttpUnauthorizedResult();
}
if (username != null && username != "")
{
bool isPermitted = false;
var viewPermission = db.RolePermissions.Where(x => x.Role == role && x.Tag == tag).SingleOrDefault();
if (viewPermission != null)
{
isPermitted = true;
}
if (isPermitted == false)
{
filterContext.Result = new RedirectToRouteResult(
new RouteValueDictionary
{
{ "controller", "Home" },
{ "action", "AccessDenied" }
});
}
}
}
- If you use [AuthorizationFilter] on top of any view then it will check this users role permitted for this view or not?. If not then it will redirect to AccessDenied page.
- Now provide the access for a Role to a View in “RolePermission” Table. [for better understand see the video]
- Run the project [see videos for more clarification]