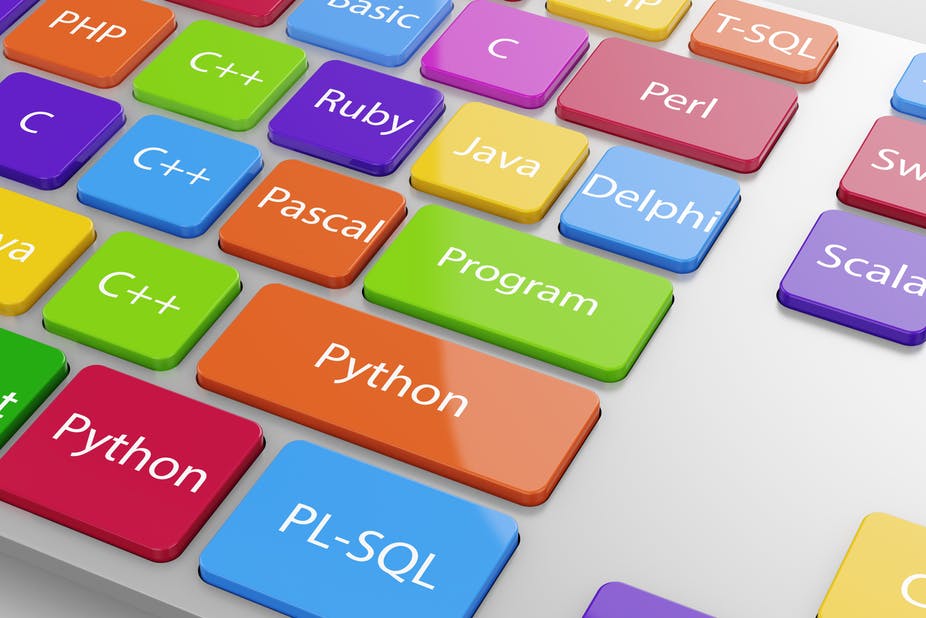
File Handling: File handling is a features of C# programming. We can create a file by the C# coding into our specific location. This file handling uses the using System.IO namespace. Using this namespace, we can create, read, write, append etc. Also, we can check the file if Exists. File handling is a import for C# or other programming language. Now given bellow the some predefine method for file handling in C#.
• Exists.
• Copy().
• Delete().
• ReadAllText()
• Flush()
• Close(). Etc.
This method uses the File class by using System.IO namespace. Now given bellow the example code and explain the code:
using System;
using System.Collections;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Reflection;
using testForClass1;
using System.IO;
namespace testFor
{
public class Program
{
static void Main(string[] args)
{
StreamWriter sw = new StreamWriter("F://jesy.txt");
Console.WriteLine("Enter the Text : ");
string str = Console.ReadLine();
sw.WriteLine(str);
sw.Flush();
sw.Close();
Console.Read();
}
}
}
First, we create a file into our F drive name as jesy.txt. This file is a text file now we create StreamWriter object name as sw. This class use the System.IO namespace. Now given the message to user write the text. Now we store the value and WriteLine method to write the text in our file. Now we use the Flush method and close method to close the StreamWriter.