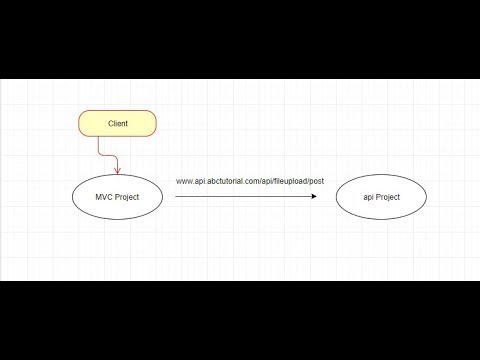
Step 1:
File saving and other data saving process is same through API so here we are saving file data but instead of file we can save any data.
Now we are creating MVC web project. We will upload and post file data from this project to API project.
- Create new project
- Create “Index” Action method.
public ActionResult Index()
{
return View();
}
- Create Index.cshtml view and paste below code.
@{
ViewBag.Title = "Home Page";
}
<div class="jumbotron">
@using (Html.BeginForm("Index", "Home", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
<table border="0" cellpadding="0" cellspacing="0">
<tr>
<td>File Attachment:</td>
<td><input type="file" name="file" multiple="multiple" /></td>
</tr>
<tr>
<td></td>
<td><input type="submit" value="Send" /></td>
</tr>
</table>
}
</div>
- Create another post Index method and code is given below
[HttpPost]
public ActionResult Index(HttpPostedFileBase file)
{
using (var client = new HttpClient())
{
using (var content = new MultipartFormDataContent())
{
MemoryStream target = new MemoryStream();
file.InputStream.CopyTo(target);
byte[] Bytes = target.ToArray();
file.InputStream.Read(Bytes, 0, Bytes.Length);
var fileContent = new ByteArrayContent(Bytes);
fileContent.Headers.ContentDisposition = new System.Net.Http.Headers.ContentDispositionHeaderValue("attachment") { FileName = file.FileName };
content.Add(fileContent);
content.Add(new StringContent("123"), "FileId");
//content.Headers.Add("Key", "abc23sdflsdf");
var requestUri = "http://localhost:65440/api/Values/";
var result = client.PostAsync(requestUri, content).Result;
if (result.StatusCode == System.Net.HttpStatusCode.Created)
{
ViewBag.Success = result.ReasonPhrase;
}
else
{
ViewBag.Failed = "Failed !" + result.Content.ToString();
}
}
}
return View();
}
Step 2:
File saving and other data saving process is same through API so here we are saving file data but instead of file we can save any data.
Now we are creating API project. We will receive file data from MVC and save using this API.
- Create API project
- Create API method name Post code is given below
public HttpResponseMessage Post()
{
var httpRequest = HttpContext.Current.Request;
if (httpRequest.Files.Count < 1)
{
return Request.CreateResponse(HttpStatusCode.BadRequest);
}
var name = httpRequest.Params["FileId"];
foreach (string file in httpRequest.Files)
{
var postedFile = httpRequest.Files[file];
var filePath = HttpContext.Current.Server.MapPath("~/" + postedFile.FileName);
postedFile.SaveAs(filePath);
}
return Request.CreateResponse(HttpStatusCode.Created);
}
- Now run Web project and API project then try to save
If you failed to save or facing any error please post your problem https://abctutorial.com/community