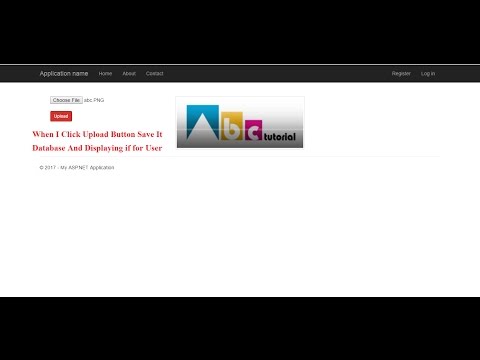
Step 1 : Create a database table
Step 2 : Click on visual studio create a new project
Step 3 : Connect your database
Step 4 : Create a new class
public class ImageViewModel
{
public int ImageId { get; set; }
public string ImageTitle { get; set; }
public byte[] ImageByte { get; set; }
public string ImagePath { get; set; }
public HttpPostedFileWrapper ImageFile { get; set; }
}
Step 5 : Create a controller and write this below code.
public class HomeController : Controller
{
public ActionResult Index()
{
MVCTutorialEntities db = new MVCTutorialEntities();
return View();
}
public JsonResult ImageUpload(ImageViewModel model)
{
MVCTutorialEntities db = new MVCTutorialEntities();
int imgId = 0;
var file = model.ImageFile;
byte[] imagebyte = null;
if (file != null)
{
file.SaveAs(Server.MapPath("/UploadImage/"+file.FileName));
BinaryReader reader = new BinaryReader(file.InputStream);
imagebyte = reader.ReadBytes(file.ContentLength);
StoreImage img = new StoreImage();
img.ImageTitle = file.FileName;
img.ImageByte = imagebyte;
img.ImagePath = "/UploadImage/" + file.FileName;
db.StoreImages.Add(img);
db.SaveChanges();
imgId = img.ImageId;
}
return Json(imgId, JsonRequestBehavior.AllowGet);
}
public ActionResult DisplayingImage(int imgid)
{
MVCTutorialEntities db = new MVCTutorialEntities();
var img = db.StoreImages.SingleOrDefault(x => x.ImageId == imgid);
return File(img.ImageByte, "image/jpg");
}
Step 6: Create a view and write this below code.
@model UploadImageInDataBase.Models.ImageViewModel
<br /><br />
<div class="container">
<div class="col-md-4">
<input type="file" id="SelectImage" /><br />
<a href="#" class="btn btn-sm btn-danger" onclick="UploadImage()">Upload</a>
</div>
<div class="col-md-4 thumbnail" id="UploadedImage"></div>
</div>
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script>
var UploadImage = function () {
var file = $("#SelectImage").get(0).files;
var data = new FormData;
data.append("ImageFile", file[0]);
$.ajax({
type: "POST",
url: "/Home/ImageUpload",
data: data,
contentType: false,
processData: false,
success: function (imgID) {
$("#UploadedImage").append('<img src="/Home/DisplayingImage?imgID=' + imgID + '"class=img-responsive thimbnail"/>');
}
})
}
</script>
Now run this application