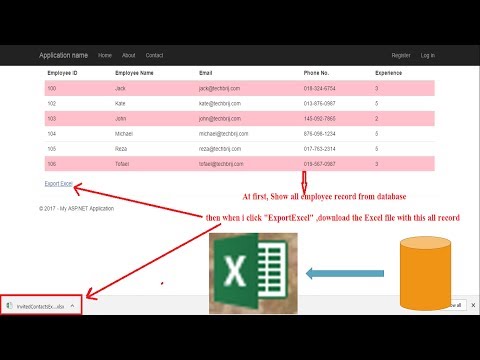
Step 1: create database.
Step 2: click on visual studio and create new project.
Step 3: install needed reference file ( EPPlus ) like as video.
Step 4 : connect your database.
Step 5 : Create a new class (EmployeeViewModel.cs).
public class EmployeeViewModel
{
public int EmployeeId { get; set; }
public string EmployeeName { get; set; }
public string Email { get; set; }
public string Phone { get; set; }
public int Experience { get; set; }
}
Step 5: create a controller and add view ( view for show the all record from the database table).
HomeController.cs
public class HomeController : Controller
{
MVCTutorialEntities db = new MVCTutorialEntities();
public ActionResult Index()
{
List<EmployeeViewModel> emplist = db.EmployeeInfoes.Select(x => new EmployeeViewModel
{
EmployeeId = x.EmployeeId,
EmployeeName = x.EmployeeName,
Email = x.Email,
Phone = x.Phone,
Experience = x.Experience
}).ToList();
return View(emplist);
}
}
Index.cshtml
@model IEnumerable<ExportExcelDemo.Models.EmployeeViewModel>
<div class="container">
<table class="table table-responsive">
<thead>
<tr>
<th>EmployeeId</th>
<th>EmployeeName</th>
<th>Email</th>
<th>Phone</th>
<th>Experience</th>
</tr>
</thead>
<tbody>
@if (Model != null)
{
foreach (var item in Model)
{
if (item.Experience < 5)
{
<tr style="background-color:pink">
<td>@item.EmployeeId</td>
<td>@item.EmployeeName</td>
<td>@item.Phone</td>
<td>@item.Email</td>
<td>@item.Experience</td>
</tr>
}
else
{
<tr>
<td>@item.EmployeeId</td>
<td>@item.EmployeeName</td>
<td>@item.Phone</td>
<td>@item.Email</td>
<td>@item.Experience</td>
</tr>
}
}
}
</tbody>
</table>
<a href="@Url.Action("ExportToExcel","Home")">Export Excel</a>
</div>
Step 6 : add new method in your controller (method for download excel file with all record).
public void ExportToExcel()
{
List<EmployeeViewModel> emplist = db.EmployeeInfoes.Select(x => new EmployeeViewModel
{
EmployeeId = x.EmployeeId,
EmployeeName = x.EmployeeName,
Email = x.Email,
Phone = x.Phone,
Experience = x.Experience
}).ToList();
ExcelPackage pck = new ExcelPackage();
ExcelWorksheet ws = pck.Workbook.Worksheets.Add("Report");
ws.Cells["A1"].Value = "Communication";
ws.Cells["B1"].Value = "Com1";
ws.Cells["A2"].Value = "Report";
ws.Cells["B2"].Value = "Report1";
ws.Cells["A3"].Value = "Date";
ws.Cells["B3"].Value = string.Format("{0:dd MMMM yyyy} at {0:H: mm tt}",DateTimeOffset.Now);
ws.Cells["A6"].Value = "EmployeeId";
ws.Cells["B6"].Value = "EmployeeName";
ws.Cells["C6"].Value = "Email";
ws.Cells["D6"].Value = "Phone";
ws.Cells["E6"].Value = "Experience";
int rowStart = 7;
foreach (var item in emplist)
{
if (item.Experience < 5)
{
ws.Row(rowStart).Style.Fill.PatternType = OfficeOpenXml.Style.ExcelFillStyle.Solid;
ws.Row(rowStart).Style.Fill.BackgroundColor.SetColor(ColorTranslator.FromHtml(string.Format("pink")));
}
ws.Cells[string.Format("A{0}", rowStart)].Value = item.EmployeeId;
ws.Cells[string.Format("B{0}", rowStart)].Value = item.EmployeeName;
ws.Cells[string.Format("C{0}", rowStart)].Value = item.Email;
ws.Cells[string.Format("D{0}", rowStart)].Value = item.Phone;
ws.Cells[string.Format("E{0}", rowStart)].Value = item.Experience;
rowStart++;
}
ws.Cells["A:AZ"].AutoFitColumns();
Response.Clear();
Response.ContentType = "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet";
Response.AddHeader("content-disposition", "attachment: filename=" + "ExcelReport.xlsx");
Response.BinaryWrite(pck.GetAsByteArray());
Response.End();
}
Now run your Application