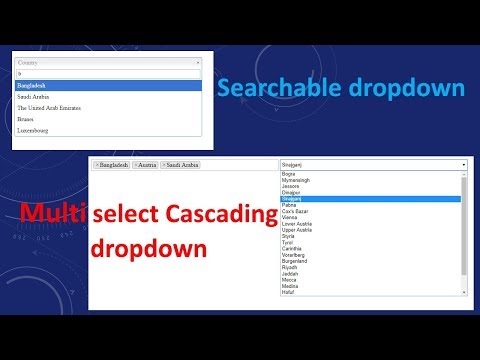
Download Project (Full Project)
Step-1
In this tutorial we create multi select cascading dropdown list. First open visual studio then create ASP.NET MVC project, give the name of the project and also you can change your solution name then click ok. Select MVC template and click ok. It’s create a project for you. It’s called default project.
Step-2
Now Go to “NuGet package manager” and install “Select2” plugin. Create a data folder and add ADO.NET Entity Framework.
This ADO.NET create to connection with SQL Sarver to fetch the data. It also create .demx file to see the table diagram.
Step-3
Given Bellow the Controller code:
using MultiSelectCascading.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MultiSelectCascading.Controllers
{
public class HomeController : Controller
{
MVCTutorialEntities db = new MVCTutorialEntities();
public ActionResult Index()
{
return View();
}
public JsonResult GetCountryList(string searchTerm)
{
var CounrtyList = db.Countries.ToList();
if (searchTerm != null)
{
CounrtyList = db.Countries.Where(x => x.CountryName.Contains(searchTerm)).ToList();
}
var modifiedData = CounrtyList.Select(x => new
{
id = x.CountryId,
text = x.CountryName
});
return Json(modifiedData, JsonRequestBehavior.AllowGet);
}
public JsonResult GetStateList(string CountryIDs)
{
List<int> CountryIdList = new List<int>();
CountryIdList = CountryIDs.Split(",".ToCharArray(), StringSplitOptions.RemoveEmptyEntries).Select(int.Parse).ToList();
List<State> StateList = new List<State>();
foreach (int countryID in CountryIdList)
{
db.Configuration.ProxyCreationEnabled = false;
var listDataByCountryID = db.States.Where(x => x.CountryId == countryID).ToList();
foreach (var item in listDataByCountryID)
{
StateList.Add(item);
}
}
return Json(StateList, JsonRequestBehavior.AllowGet);
}
}
}
Step-4
Given Bellow the index.cshtml view code:
@{
Layout = null;
}
<link href="~/Content/css/select2.min.css" rel="stylesheet" />
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script src="~/Scripts/select2.min.js"></script>
<br /><br />
<input type="hidden" class="CountryId"/>
<select class="Country" style="width:500px" multiple>
</select>
<select class="State" style="width:500px;height:30px;border-radius:5px;" >
<option>Select State</option>
</select>
<script>
$(document).ready(function () {
$(".Country").select2({
placeholder: "Counrty",
theme: "classic",
ajax: {
url: "/Home/GetCountryList",
dataType: "json",
data: function (params) {
return {
searchTerm: params.term
};
},
processResults: function (data, params) {
return {
results: data
};
}
}
});
});
$(".Country").on("change", function () {
var CounrtyID = $(this).val();
$(".CountryId").val(CounrtyID);
var textBoxVal = $(".CountryId").val();
$.ajax({
url: "/Home/GetStateList?CountryIDs=" + textBoxVal,
dataType: 'json',
type: 'post',
success: function (data) {
$(".State").empty();
$.each(data, function (index, row) {
$(".State").append("<option value='" + row.StateId + "'>" + row.StateName + "</option>")
});
}
});
});
</script>
Step-5
Now run the project.