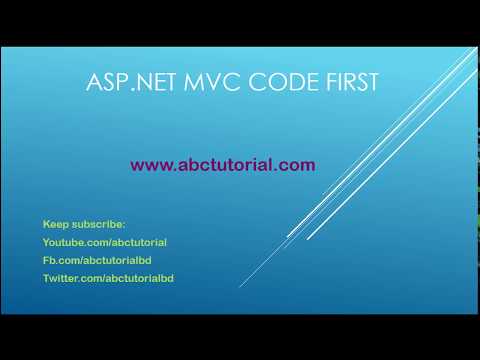
Download Project (Full Project)
Step 1:
In this tutorial I will show how to work Entity Framework Code First ASP.NET MVC. Open visual studio then create ASPNET MVC web application. Now create two models, this model are create the database. This model property is going to table column and this file name is column name. Then create file for DbContext. This Dbcontext inherit the my context file. Given bellow the model code with relation:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace CodeFirst.Models
{
public class Student
{
public Student()
{
StudentSubjectTakens = new List<StudentSubjectTaken>();
}
public int Id { get; set; }
public string Name { get; set; }
public virtual ICollection<StudentSubjectTaken> StudentSubjectTakens { get; set; }
}
}
Now given bellow another model code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace CodeFirst.Models
{
public class StudentSubjectTaken
{
public StudentSubjectTaken()
{
}
public int Id { get; set; }
public int StudentId { get; set; }
public string SubjectCode { get; set; }
public Student Student { get; set; }
}
}
Step 2:
Context is responsible to create database and connect database server. This context class inherits the DbContext class. Given bellow the code:
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Linq;
using System.Web;
namespace CodeFirst.Models
{
public partial class CodeFContext:DbContext
{
public CodeFContext()
: base("Name=MyContext")
{
}
public DbSet<Student> Students { get; set; }
public DbSet<StudentSubjectTaken> StudentSubjectTakens { get; set; }
}
}
Step 3:
Now Home controller gets the database value and returns the view by return key word. Also include the Student table and pass the view. Given bellow the controller code:
using CodeFirst.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace CodeFirst.Controllers
{
public class HomeController : Controller
{
CodeFContext context = new CodeFContext();
public ActionResult Index()
{
var data = context.StudentSubjectTakens.Include("Student").ToList();
return View(data);
}
public ActionResult About()
{
ViewBag.Message = "Your application description page.";
return View();
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
}
}
Step 4:
Now view is represent the value by the foreach loop and also the value get by model. Now given bellow the view code:
@model IEnumerable<CodeFirst.Models.StudentSubjectTaken>
@{
ViewBag.Title = "Home Page";
}
<div class="row">
@foreach (var item in Model)
{
<p>Name:@item.Student.Name Code:@item.SubjectCode</p>
}
</div>
Step 5:
Now build and run the project.