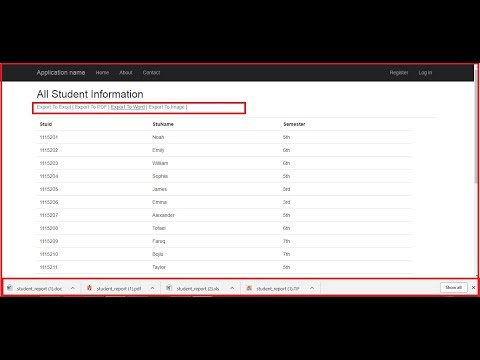
Step 1:
In this tutorial I will show how to Export Database Data in PDF | Word | Excel And Image File | RDLC Report in MVC. First open visual studio. Then create an asp.net web application. Then select MVC and click ok. Visual studio creates a default project for you. Now add ado.net entity data model for fetch the data from database. And this data pass the view. In this view create some link tag using rezor. This link tag is responsible to tiger the reports method and passes the perimeter like PDF, JPG, EXCEL etc. This view also shows the list of student by the “foreeach” loop using student model. Given bellow the view code:
@model IEnumerable<HighChart.Data.Student>
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
<p>
@Html.ActionLink("Download PDF","Reports",new {ReportType= "PDF" }) |
@Html.ActionLink("Download Word" , "Reports", new {ReportType = "Word"} ) |
@Html.ActionLink("Download Excel", "Reports", new { ReportType = "Excel" }) |
@Html.ActionLink("Download Jpg", "Reports", new { ReportType = "JPG" }) |
</p>
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.Roll)
</th>
<th>
@Html.DisplayNameFor(model => model.AccountID)
</th>
<th>
@Html.DisplayNameFor(model => model.Batch)
</th>
<th>
@Html.DisplayNameFor(model => model.Semester)
</th>
<th>
@Html.DisplayNameFor(model => model.Dept)
</th>
<th>
@Html.DisplayNameFor(model => model.Sex)
</th>
<th>
@Html.DisplayNameFor(model => model.StdMobile)
</th>
<th>
@Html.DisplayNameFor(model => model.SSCresult)
</th>
<th>
@Html.DisplayNameFor(model => model.HSCresult)
</th>
<th>
@Html.DisplayNameFor(model => model.DOB)
</th>
<th>
@Html.DisplayNameFor(model => model.Picture)
</th>
<th>
@Html.DisplayNameFor(model => model.FathersName)
</th>
<th>
@Html.DisplayNameFor(model => model.FathersMobile)
</th>
<th>
@Html.DisplayNameFor(model => model.MothersName)
</th>
<th>
@Html.DisplayNameFor(model => model.MothersMobile)
</th>
<th>
@Html.DisplayNameFor(model => model.FathersProfession)
</th>
<th>
@Html.DisplayNameFor(model => model.MothersProfession)
</th>
<th>
@Html.DisplayNameFor(model => model.CurrGaredian)
</th>
<th>
@Html.DisplayNameFor(model => model.CurrGaredianMobile)
</th>
<th>
@Html.DisplayNameFor(model => model.CurrGaredianAddress)
</th>
<th>
@Html.DisplayNameFor(model => model.Status)
</th>
<th>
@Html.DisplayNameFor(model => model.PresentAddress)
</th>
<th>
@Html.DisplayNameFor(model => model.ParmanentAddress)
</th>
<th>
@Html.DisplayNameFor(model => model.Email)
</th>
<th>
@Html.DisplayNameFor(model => model.UserName)
</th>
<th>
@Html.DisplayNameFor(model => model.Password)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.Roll)
</td>
<td>
@Html.DisplayFor(modelItem => item.AccountID)
</td>
<td>
@Html.DisplayFor(modelItem => item.Batch)
</td>
<td>
@Html.DisplayFor(modelItem => item.Semester)
</td>
<td>
@Html.DisplayFor(modelItem => item.Dept)
</td>
<td>
@Html.DisplayFor(modelItem => item.Sex)
</td>
<td>
@Html.DisplayFor(modelItem => item.StdMobile)
</td>
<td>
@Html.DisplayFor(modelItem => item.SSCresult)
</td>
<td>
@Html.DisplayFor(modelItem => item.HSCresult)
</td>
<td>
@Html.DisplayFor(modelItem => item.DOB)
</td>
<td>
@Html.DisplayFor(modelItem => item.Picture)
</td>
<td>
@Html.DisplayFor(modelItem => item.FathersName)
</td>
<td>
@Html.DisplayFor(modelItem => item.FathersMobile)
</td>
<td>
@Html.DisplayFor(modelItem => item.MothersName)
</td>
<td>
@Html.DisplayFor(modelItem => item.MothersMobile)
</td>
<td>
@Html.DisplayFor(modelItem => item.FathersProfession)
</td>
<td>
@Html.DisplayFor(modelItem => item.MothersProfession)
</td>
<td>
@Html.DisplayFor(modelItem => item.CurrGaredian)
</td>
<td>
@Html.DisplayFor(modelItem => item.CurrGaredianMobile)
</td>
<td>
@Html.DisplayFor(modelItem => item.CurrGaredianAddress)
</td>
<td>
@Html.DisplayFor(modelItem => item.Status)
</td>
<td>
@Html.DisplayFor(modelItem => item.PresentAddress)
</td>
<td>
@Html.DisplayFor(modelItem => item.ParmanentAddress)
</td>
<td>
@Html.DisplayFor(modelItem => item.Email)
</td>
<td>
@Html.DisplayFor(modelItem => item.UserName)
</td>
<td>
@Html.DisplayFor(modelItem => item.Password)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.ID }) |
@Html.ActionLink("Details", "Details", new { id=item.ID }) |
@Html.ActionLink("Delete", "Delete", new { id=item.ID })
</td>
</tr>
}
</table>
Step 2:
Now index controller pass the get the data from database and pass the view and Reports controller take the one parameter name as “ReportType” this parameter take one string type value and check by the if else condition and export the report help of RDLC report. Given bellow the controller code:
using HighChart.Data;
using Microsoft.Reporting.WebForms;
using Microsoft.Reporting.WebForms.Internal.Soap.ReportingServices2005.Execution;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace HighChart.Controllers
{
public class ExportDatabaseController : Controller
{
SRMSDbContext db = new SRMSDbContext();
// GET: ExportDatabase
public ActionResult Index()
{
var std =db.Student.ToList();
return View(std.ToList());
}
public ActionResult Reports(string ReportType)
{
LocalReport localReport = new LocalReport();
localReport.ReportPath= Server.MapPath("~/Reports/StudentReport.rdlc");
ReportDataSource reportDataSource = new ReportDataSource();
reportDataSource.Name = "StudentDataSet";
reportDataSource.Value = db.Student.ToList();
localReport.DataSources.Add(reportDataSource);
string reportType = ReportType;
string mimeTime;
string encoding;
string fileNameExtrention;
if (reportType=="Excel")
{
fileNameExtrention = "xlsx";
}
else if (reportType == "Word")
{
fileNameExtrention = "docsx";
}
else if (reportType == "PDF")
{
fileNameExtrention = "pdf";
}
else
{
fileNameExtrention = "jpg";
}
string[] streams;
Microsoft.Reporting.WebForms.Warning[] warnings;
byte[] renderdByte;
renderdByte = localReport.Render(reportType, "", out mimeTime, out encoding, out fileNameExtrention, out streams, out warnings);
Response.AddHeader("Content Description","Attesment;fileName=studentReport."+fileNameExtrention);
return File(renderdByte,fileNameExtrention);
}
}
}
Step 3:
Now build and run the project.