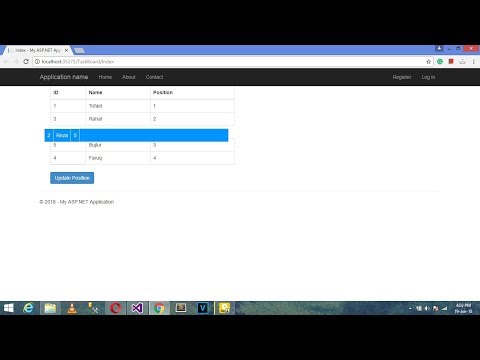
Step 1:
In this tutorial I will show how to create jquery Drag & Drop In ASP.NET MVC. First open visual studio and create a ASP.NET Web Application and select MVC. Now create database in SQL server management studio. Now this database connects to the project by the ADO.NET Entity framework. When we connect the database to our project we see the edmx file. Now save the file and build the project. In the controller we have two method. “Index” method just gets the data and passes the view. And “UpdateItem” method takes the parameter and find and sort by the list and pass the view and update the database. Given bellow the controller code:
using HighChart.Data;
using System;
using System.Collections.Generic;
using System.Data.Entity.Migrations;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace HighChart.Controllers
{
public class SortRowController : Controller
{
crudEntities context = new crudEntities();
public ActionResult Index()
{
var item = context.Post.ToList();
var item2 = item.OrderBy(x => x.RowNo);
return View(item2.ToList());
}
public ActionResult UpdateItem(string itemIds)
{
int count = 1;
List<int> itemIdList = new List<int>();
itemIdList = itemIds.Split(",".ToArray(), StringSplitOptions.RemoveEmptyEntries).Select(int.Parse).ToList();
foreach (var itemId in itemIdList)
{
try
{
Post item = context.Post.Where(x => x.Id == itemId).FirstOrDefault();
item.RowNo = count;
context.Post.AddOrUpdate(item);
context.SaveChanges();
}
catch (Exception)
{
continue;
}
count++;
}
return Json(true,JsonRequestBehavior.AllowGet);
}
}
}
Step 2:
View is representing the data by help of the model and we use this view jquery-ui for sort the data by drag and drop. We also use ajax post for the update method called. We can also show the data on the view by using “foreach” loop and also table tag use in this view. Now given bellow the view code:
@model IEnumerable<HighChart.Data.Post>
@{
ViewBag.Title = "Index";
}
<script src="~/Scripts/jquery-ui-1.12.1.min.js"></script>
<script>
$(document).ready(function () {
$("#sortable").sortable({
update: function (event, ui) {
var itemIds = "";
$("#sortable").find(".taskSingleLine").each(function () {
var itemId = $(this).attr("data-taskid");
itemIds = itemIds + itemId + ",";
});
$.ajax({
url: '@Url.Action("UpdateItem", "SortRow")',
data: { itemIds: itemIds },
type: 'POST',
success: function (data) {
},
error: function (xhr,status,error) {
}
});
}
})
})
</script>
<style>
#sortable tr:hover{
background-color:green;
color:white;
}
</style>
<h2>Index</h2>
<div class="container">
<div class="col-md-6">
<table class="table table-bordered">
<thead>
<tr>
<td>ID</td>
<td>Name</td>
<td>Position</td>
</tr>
</thead>
<tbody id="sortable" style="cursor:pointer">
@foreach (var item in Model)
{
<tr>
<td>@item.Id</td>
<td class="taskSingleLine" id="task@(item.Id)" data-taskid="@(item.Id)">@item.Name</td>
<td>@item.RowNo</td>
</tr>
}
</tbody>
</table>
<a class="btn btn-success" href="@Url.Action("Index","SortRow")">Update Position</a>
</div>
</div>
Step 3:
We must and should install “Jquery-ui” from “nuGet” Package manager. Now build and run the project.