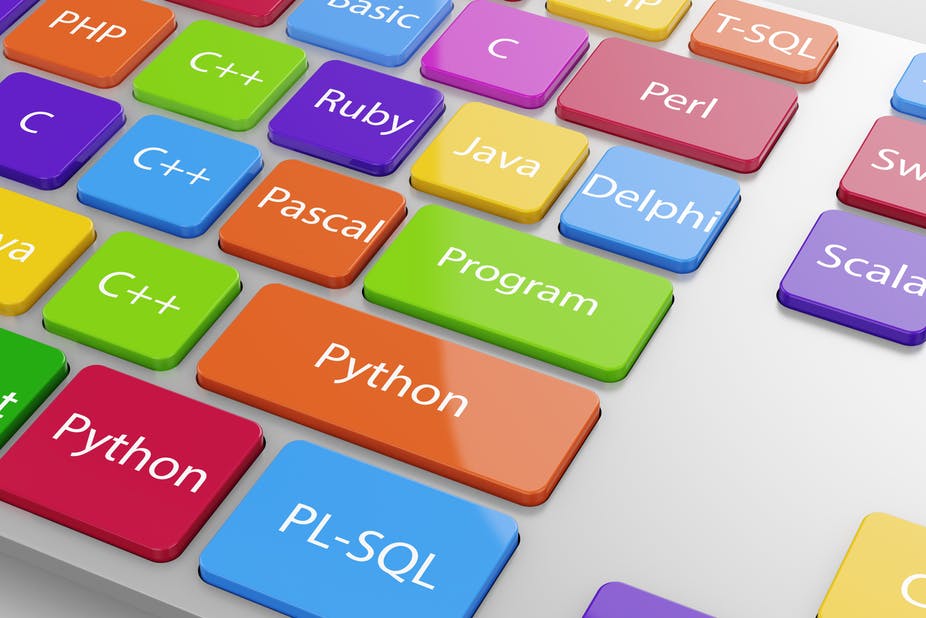
JavaScript ES5 introduce some method for using our object to find, search, sort, replace the under-object property value. This object method is very help full for programmer. When we create an object then we can change the value of the specific property from the object then we use the method and very easily we can do it. Now given bellow some object method name:
// Adding or changing an object property
Object.defineProperty(object, property, descriptor)
// Adding or changing many object properties
Object.defineProperties(object, descriptors)
// Accessing Properties
Object.getOwnPropertyDescriptor(object, property)
// Returns all properties as an array
Object.getOwnPropertyNames(object)
// Returns enumerable properties as an array
Object.keys(object)
// Accessing the prototype
Object.getPrototypeOf(object)
// Prevents adding properties to an object
Object.preventExtensions(object)
// Returns true if properties can be added to an object
Object.isExtensible(object)
// Prevents changes of object properties (not values)
Object.seal(object)
// Returns true if object is sealed
Object.isSealed(object)
// Prevents any changes to an object
Object.freeze(object)
// Returns true if object is frozen
Object.isFrozen(object)
Now given bellow the object method example code and explain the code:
<!DOCTYPE html>
<html>
<head>
<title></title>
</head>
<body>
<script type="text/javascript">
//JavaScript object method
var human = {
firstName: "Reza",
lastName : "Karim",
language : "EN"
};
// Change a property
Object.defineProperty(human, "language", {value:"Bang"})
//print the changed value
console.log(human.language);
</script>
</body>
</html>
In this code we show the one object method example code how to work. First create an object name as ‘human’ then this object contain three property like ‘firstName’, ‘lastName’, ‘language’ then assign the default value of the object. Now we use the object method change the language value also print the language value using ‘console.log’.