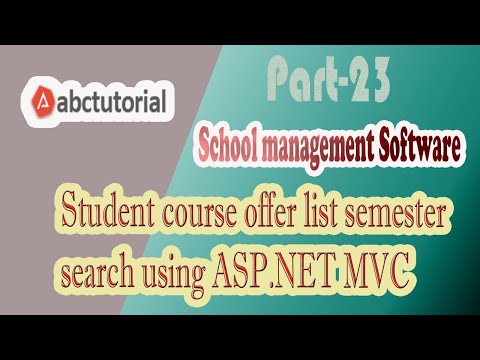
In this post, I would like to Part 23 Student Course Offer List Student ID No and Trimester Search to get Course Info using Jquery & Angular js in ASP.NET MVC.
Step-1: In StudentCourseOfferController.cs Class Add GetStudentCourseOfferList() for load Student Course Offer List.
Go to Solution Explorer > Controllers Folder > StudentCourseOfferController.css with writing the below code.
public ActionResult GetStudentCourseOfferList(string prm)
{
DataTable dr = aDal.GetStudentCourseOfferListDAL(prm);
string jJSONresult;
jJSONresult = JsonConvert.SerializeObject(dr);
return Json(jJSONresult, JsonRequestBehavior.AllowGet);
}
Step-2: In StudentCourseOfferDAL.cs Class Add GetStudentCourseOfferListDAL() for load Student Course Offer List.
Go to Solution Explorer > DAL Folder > StudentCourseOfferDAL.css with writing the below code
public DataTable GetStudentCourseOfferListDAL(string prm)
{
SqlCommand com = new SqlCommand("sp_StudentCourseOfferListLoadByParm", conn);
com.CommandType = CommandType.StoredProcedure;
com.Parameters.AddWithValue("@prm", prm);
SqlDataAdapter da = new SqlDataAdapter(com);
DataTable dss = new DataTable();
da.Fill(dss);
return dss;
}
Step-3: Create store Procedure for Save Student Course Offer List.
Go to SQL Server 2014 > dbStudentMangeSystem database> Programmability> stored procedures> Select New> stored procedure>Create sp_StudentCourseOfferListLoadByParm
with writing the below code
create proc [dbo].[sp_StudentCourseOfferListLoadByParm]
@prm nvarchar(max)
as
begin
Declare @Query nvarchar(max)
set @Query ='select co.CourseName, * from tblStudentCourseOffer
left join tblCourse co on tblStudentCourseOffer.CourseId=co.CourseId
where StudentCourseOfferId is not null '+@prm
end
exec(@Query)
Step-4: search button click to get Student Course Offer List by select Student ID and Trimester Name StudentCourseOfferList page with writing the highlight code.
<script>
function GetList() {
var prm = "";
if ($('#StudentId').val() != "") {
prm = prm + " and StudentId ='" + $('#StudentId').val() + "'";
}
if ($('#TrimesterInfoId').val() != "") {
prm = prm + " and TrimesterInfoId ='" + $('#TrimesterInfoId').val() + "'";
}
var urlPath = '@Url.Action("GetStudentCourseOfferList", "StudentCourseOffer")';
$.ajax({
url: urlPath,
dataType: 'json',
data: { prm: prm},
type: "Get",
async: true,
success: function (data) {
var result = JSON.parse(data);
var row = "";
$('#dtTableBody').html("");
for (var i = 0; i < result.length;i++) {
row +="<tr>";
row += "<td>" + (i + 1) + "</td>";
row += "<td>" + result[i].CourseName + "</td>";
row += "</tr>";
}
$('#dtTableBody').html(row);
},
error: function (data) {
alert("Operation Faild!!");
}
})
}
</script>
Step-5: Run Application.