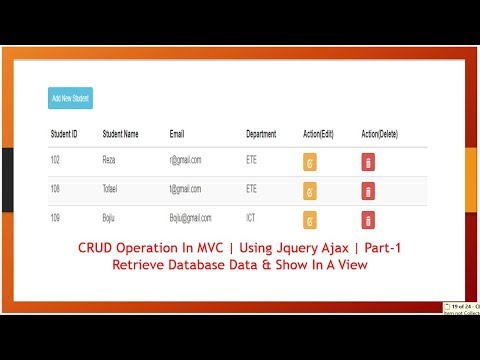
CRUD Operations In MVC Using Jquery Ajax | Part-2 Create PopUp Modal With Registration Form
CRUD Operation In MVC Using Jquery Ajax | Part-1 Retrieve Database Data & Show In A View
CRUD Operations In MVC Using Jquery Ajax | Part-3 Data Save,Update & Delete In Database
Download Project (Full Project)
Step-1 : Create A Database Table
"tblDepartment"
"tblStudent"
Step-2 : Click On Visual Studio Create A New Project
- File”>New>Project>Visual c#>Web> then provide your project name> then select “ok”> then click “MVC”>ok.
Step 3 : Connect Your Database Using Entity Framework.
- Right click on folder named “Model”>Add>New Item>ADO.NET Entity DataModel>ok>Generate from database>next>New connection>Provide your SQL Server name to “Server name” textfield>Select database from “database name”>ok>next
- Expand “table”>expand “dbo”>select your created table>“finish (If You Do Not Understood This Please Watch Our Video)
Step 4 : Create A New Model Class (Like As Video).
"StudentViewModel"
public class StudentViewModel
{
public int StudentId { get; set; }
public string StudentName { get; set; }
public string Email { get; set; }
public Nullable<bool> IsDeleted { get; set; }
public Nullable<int> DepartmentId { get; set; }
public string DepartmentName { get; set; }
}
Step 5 : Write Down The Below Code In Your Controller
"HomeController"
public class HomeController : Controller
{
MVCTutorialEntities db = new MVCTutorialEntities();
public ActionResult Index()
{
//Pass All Department List Using ViewBag
List<tblDepartment> DeptList = db.tblDepartments.ToList();
ViewBag.ListOfDepartment = new SelectList(DeptList, "DepartmentId", "DepartmentName");
return View();
}
public JsonResult GetStudentList()
{
//Pass The All Student Record From Controller To View For Show The All Data For User
List<StudentViewModel> StuList = db.tblStudents.Where(x => x.IsDeleted == false).Select(x => new StudentViewModel
{
StudentId = x.StudentId,
StudentName = x.StudentName,
Email = x.Email,
DepartmentName = x.tblDepartment.DepartmentName
}).ToList();
return Json(StuList, JsonRequestBehavior.AllowGet);
}
}
Step 6 : Add A View And Write Down The Below Code In Your View
"Index.cshtml"
@model FullCRUDImplementationWithJquery.Models.StudentViewModel
@{
Layout = null;
}
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script src="~/Scripts/bootstrap.min.js"></script>
<link href="~/Content/bootstrap.min.css" rel="stylesheet" />
<div class="container" style="margin-top:3%">
<a href="#" class="btn btn-info" onclick="AddNewStudent(0)">Add New Student</a> <br /><br />
<table class="table table-striped">
<thead>
<tr>
<th>Student ID</th>
<th>Student Name</th>
<th>Email</th>
<th>Department</th>
<th>Action(Edit)</th>
<th>Action(Delete)</th>
</tr>
</thead>
<tbody id="SetStudentList">
<tr id="LoadingStatus" style="color:red"></tr>
</tbody>
</table>
</div>
<script>
$("#LoadingStatus").html("Loading....");
$.get("/Home/GetStudentList", null, DataBind);
function DataBind(StudentList) {
//This Code For Receive All Data From Controller And Show It In Client Side
var SetData = $("#SetStudentList");
for (var i = 0; i < StudentList.length; i++) {
var Data = "<tr class='row_" + StudentList[i].StudentId + "'>" +
"<td>" + StudentList[i].StudentId + "</td>" +
"<td>" + StudentList[i].StudentName + "</td>" +
"<td>" + StudentList[i].Email + "</td>" +
"<td>" + StudentList[i].DepartmentName + "</td>" +
"<td>" + "<a href='#' class='btn btn-warning' onclick='EditStudentRecord(" + StudentList[i].StudentId + ")' ><span class='glyphicon glyphicon-edit'></span></a>" + "</td>" +
"<td>" + "<a href='#' class='btn btn-danger' onclick='DeleteStudentRecord(" + StudentList[i].StudentId + ")'><span class='glyphicon glyphicon-trash'></span></a>" + "</td>" +
"</tr>";
SetData.append(Data);
$("#LoadingStatus").html(" ");
}
}
</script>
Run This Project
Part-1 Is Done.... Must Watch Part-2 And Part-3 For Complete This Project(Full CRUD Operation In MVC) [Click Next For Part-2]