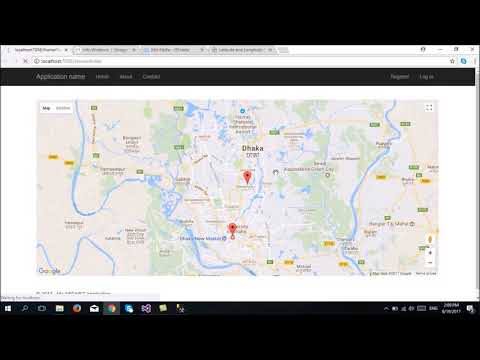
Download Project (Full Project)
Step-1
In this part i will show how to add and show marker (Location) in Google map using Jquery. Now Open visual studio then open our existing project where we are working. Then go to https://developers.google.com/ link. Then click web and click JavaScript api. In this index.cshtml file we need to add and modify some code and this code is show the marker. Also show the details of the location. Now given bellow the index.cshtml views code:
@{
ViewBag.Title = "Home Page";
}
<style>
#map {
height: 500px;
}
</style>
<br /><br />
<div id="map"></div>
<script>
var map;
function initMap() {
map = new google.maps.Map(document.getElementById('map'), {
center: { lat: 23.778074, lng: 90.397514 },
zoom: 12
});
//For Dhaka U
var markerBracU = new google.maps.Marker({
position: { lat: 23.780168, lng: 90.407191 },
map: map
});
var contentStringBracU = '<div id="content">' +
'<div id="siteNotice">' +
'</div>' +
'<h1 id="firstHeading" class="firstHeading">BracU</h1>' +
'<div id="bodyContent">' +
'<p><b>BracU</b>, also referred to as <b>Ayers Rock</b>, is a large ' +
'sandstone rock formation in the southern part of the ' +
'Northern Territory, central Australia. It lies 335 km (208 mi) ' +
'south west of the nearest large town, Alice Springs; 450 km ';
var infowindowForBracU = new google.maps.InfoWindow({
content: contentStringBracU
});
markerBracU.addListener('mouseover', function () {
infowindowForBracU.open(map, markerBracU);
});
markerBracU.addListener('mouseout', function () {
infowindowForBracU.close();
});
//For Dhaka U
var markerDhakaU = new google.maps.Marker({
position: { lat: 23.733994, lng: 90.392784 },
map: map
});
var contentStringDhakaU = '<div id="content">' +
'<div id="siteNotice">' +
'</div>' +
'<h1 id="firstHeading" class="firstHeading">DhakaU</h1>' +
'<div id="bodyContent">' +
'<p><b>DhakaU</b>, also referred to as <b>Ayers Rock</b>, is a large ' +
'sandstone rock formation in the southern part of the ' +
'Northern Territory, central Australia. It lies 335 km (208 mi) ' +
'south west of the nearest large town, Alice Springs; 450 km ';
var infowindowForDhakaU = new google.maps.InfoWindow({
content: contentStringDhakaU
});
markerDhakaU.addListener('mouseover', function () {
infowindowForDhakaU.open(map, markerDhakaU);
});
markerDhakaU.addListener('mouseout', function () {
infowindowForDhakaU.close();
});
}
</script>
<script src="https://maps.googleapis.com/maps/api/js?key=AIzaSyDJOZd-P3bAJXBAYtfryQT_GTv61uRehMs&callback=initMap" async defer></script>
Step-2
This Google Map is full JavaScript code depends. No need to write extra code into server site. Just c# method return a view and this view represent the Google map. Given bellow the controller code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace GoogleMap.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
public ActionResult About()
{
ViewBag.Message = "Your application description page.";
return View();
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
}
}
Step-3
Now build and run the project.