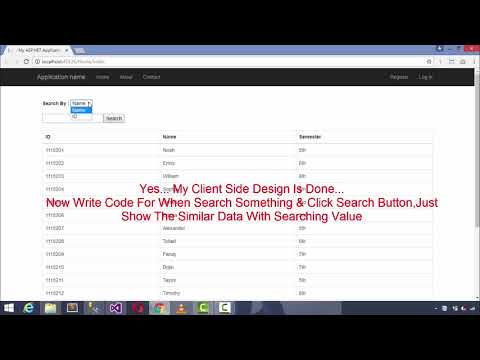
Download Project (Full Project)
Step-1
In this part i will show how to include Search Functionality in ASP.NET MVC using Jquery Ajax. First create a asp.net web application then click ok and select MVC then click ok. Visual studio create a default project for you. Then connect ADO.NET entity data model into models folder. Then build the solution. Now go to Home controller and create database object name as db. Then by the db object access the table under Home controller then Index method return the table by the return key word with list. “GetSearchingData” this method receives two parameter for search the value and return the view. Also every method handle the exception. Now given bellow the Home controller code:
using SearchFunctionalityWithJquery.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace SearchFunctionalityWithJquery.Controllers
{
public class HomeController : Controller
{
MVCTutorialEntities db = new MVCTutorialEntities();
public ActionResult Index()
{
return View(db.StudentInfoes.ToList());
}
public JsonResult GetSearchingData(string SearchBy, string SearchValue)
{
List<StudentInfo> StuList = new List<StudentInfo>();
if (SearchBy == "ID")
{
try
{
int Id = Convert.ToInt32(SearchValue);
StuList = db.StudentInfoes.Where(x => x.StuId == Id || SearchValue == null).ToList();
}
catch (FormatException)
{
Console.WriteLine("{0} Is Not A ID ", SearchValue);
}
return Json(StuList, JsonRequestBehavior.AllowGet);
}
else
{
StuList = db.StudentInfoes.Where(x => x.StuName.StartsWith(SearchValue) || SearchValue == null).ToList();
return Json(StuList, JsonRequestBehavior.AllowGet);
}
}
}
}
Step-2
Now create the index view. This view contents the ID and Name by the dropdown list. When user select the id then the text field search only id and if user select name then the search are find only name otherwise it search every field by using jQuery. First show the all table value in the view then it search the data then the particular value find and show the on the view. Now given bellow the view code:
@model IEnumerable<SearchFunctionalityWithJquery.Models.StudentInfo>
<br /><br />
<div class="container">
<b>Search By : </b>
<select id="SearchBy">
<option value="Name">Name</option>
<option value="ID">ID</option>
</select><br /><br />
@Html.TextBox("Search")<input type="submit" id="SearchBtn" value="Search" /><br /><br />
<table class="table table-bordered">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Semester</th>
</tr>
</thead>
<tbody id="DataSearching">
@foreach (var Item in Model)
{
<tr>
<td>@Item.StuId</td>
<td>@Item.StuName</td>
<td>@Item.Semester</td>
</tr>
}
</tbody>
</table>
</div>
<script src="~/Scripts/jquery-1.10.2.min.js"></script>
<script>
$(document).ready(function () {
$("#SearchBtn").click(function () {
var SearchBy = $("#SearchBy").val();
var SearchValue = $("#Search").val();
var SetData = $("#DataSearching");
SetData.html("");
$.ajax({
type: "post",
url: "/Home/GetSearchingData?SearchBy=" + SearchBy + "&SearchValue=" + SearchValue,
contentType: "html",
success: function (result) {
if (result.length == 0) {
SetData.append('<tr style="color:red"><td colspan="3">No Match Data</td></tr>')
}
else {
$.each(result, function (index, value) {
var Data = "<tr>" +
"<td>" + value.StuId + "</td>" +
"<td>" + value.StuName + "</td>" +
"<td>" + value.Semester + "</td>" +
"</tr>";
SetData.append(Data);
});
}
}
});
});
});
</script>
Step-3
Now build and run the project.