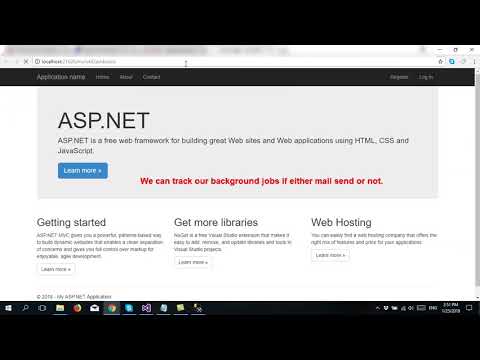
Download Project (Full Project)
Step-1:
In this part i will show how to Send Email using Hangfire Scheduler ASP.NET MVC. First Open visual studio then opens our existing project where we are working. Now write some code into home controller. Under home controller write some method like SendEmail this method send the email and EMailTemplate method get the template from content folder and attach the template and SendEmailAsync method receive three parameter and configure the smtp client property and send the mail. Now given bellow the controller code:
using Hangfire;
using System;
using System.Collections.Generic;
using System.Configuration;
using System.Globalization;
using System.Linq;
using System.Net;
using System.Net.Mail;
using System.Threading.Tasks;
using System.Web;
using System.Web.Hosting;
using System.Web.Mvc;
namespace exampleOfHangfire.Controllers
{
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
public async Task<ActionResult> SendEmail()
{
//Email
var message = EMailTemplate("WelcomeEmail");
message = message.Replace("@ViewBag.Name", CultureInfo.CurrentCulture.TextInfo.ToTitleCase("Reza"));
message = message.Replace("@ViewBag.Details", "This is Test Details");
await SendEmailAsync(toEmail, "Test subject", message);
//End Email
return Json(0,JsonRequestBehavior.AllowGet);
}
public string EMailTemplate(string template)
{
string body = System.IO.File.ReadAllText(HostingEnvironment.MapPath("~/Content/template/") + template + ".cshtml");
return body.ToString();
}
public async static Task SendEmailAsync(string email,string subject,string message)
{
try
{
var _email = "fsjesy@gmail.com";
var _epass = ConfigurationManager.AppSettings["EmailPassword"];
var _dispName = "Test Mail";
MailMessage myMessage = new MailMessage();
myMessage.To.Add(email);
myMessage.From = new MailAddress(_email, _dispName);
myMessage.Subject = subject;
myMessage.Body = message;
myMessage.IsBodyHtml = true;
using (SmtpClient smtp=new SmtpClient())
{
smtp.EnableSsl = true;
smtp.Host = "smtp.gmail.com";
smtp.Port = 587;
smtp.UseDefaultCredentials = false;
smtp.Credentials = new NetworkCredential(_email, _epass);
smtp.DeliveryMethod = SmtpDeliveryMethod.Network;
smtp.SendCompleted += (s, e) => { smtp.Dispose(); };
await smtp.SendMailAsync(myMessage);
}
}
catch (Exception)
{
throw;
}
}
}
}
Step-2:
View is nothing but represent the content. This content came from the home controller using ViewBag. Given bellow the WelcomEmail.cshtml code under templates folder:
<div>
Name: @ViewBag.Name
Details: @ViewBag.Details
</div>
Step-3:
Now open Startup.cs class and Write some configuration code for send mail. Given Bellow the code:
using exampleOfHangfire.Controllers;
using exampleOfHangfire.Models;
using Hangfire;
using Microsoft.Owin;
using Owin;
using System;
[assembly: OwinStartupAttribute(typeof(exampleOfHangfire.Startup))]
namespace exampleOfHangfire
{
public partial class Startup
{
public void Configuration(IAppBuilder app)
{
ConfigureAuth(app);
GlobalConfiguration.Configuration.UseSqlServerStorage("DefaultConnection");
app.UseHangfireDashboard("/myJobDashboard");
HomeController obj = new HomeController();
//BackgroundJob.Enqueue(() => Console.WriteLine("Fire-and-forget!"));
RecurringJob.AddOrUpdate(() => obj.SendEmail(),Cron.Minutely);
app.UseHangfireServer();
}
}
}
Step-4:
Now Under Web.Config file into AppSetting section add this line. Given bellow the code:
<add key="EmailPassword" value="true" />
Step-5:
Now build and run the project.