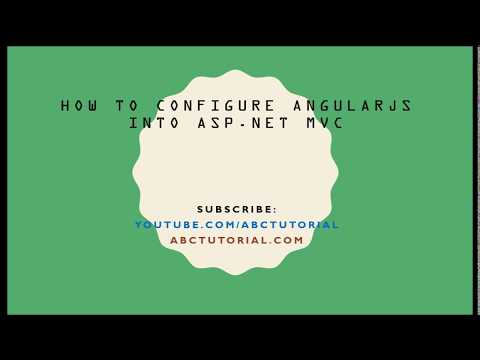
Download Project (Full Project)
Step-1
In this part i will show how to setup AngularJS into ASP.NET MVC project. First open visual studio then create new project and select asp.net web application then click ok now select MVC then click ok. Visual studio create default project for you. Now install AngularJS from Package Manager Console. Command “Install-Package angularjs” then Enter. You can see your Angularjs file into scripts folder. Now first drag and drop the Angular.min.js file into layout file under the head section. Also create a folder under scripts folder, name is customjs folder. This customjs folder contain the our won JavaScript file. Now create App.js file and write some angular code. This App.js file contain the “ng-app” directive. Given bellow the App.js file code:
var deps = [];
var app = angular.module("myApp", deps);
Step-2
Now create HomeIndexController file under custom folder. This file represents the angularjs controller. Also all logical code handle this file. Now drag and drop the “HomeIndexController” file under Home->Index.cshtml bellow the code. Also write ng-controller in this file. Given bellow the HomeIndexController.js files code:
(function () {
app.controller('TestMy', function ($scope, $http) {
$scope.GetPageData = function () {
$http.get('//Home/GetPaggedData').then(function (myData) {
$scope.adminList = myData.data;
alert("This is my value"+$scope.adminList);
});
}
}).call(angular);
Step-3
Now c# controller passes the object by json formatting. And this data receive the JavaScript controller and show the view. Given bellow the controller code:
using asp.net_mvc_pagging_angularjs.Helper;
using asp.net_mvc_pagging_angularjs.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace asp.net_mvc_pagging_angularjs.Controllers
{
public class HomeController : Controller
{
public ActionResult GetPaggedData()
{
int paggedData=10;
return Json(paggedData, JsonRequestBehavior.AllowGet);
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
}
}
Step-4
Index cshtml file just represent/display the value from angular js controller. When click the button then it call the angular function and this function call the c# method and c# method pass the json data and angularjs file receive the data and Bind the view by the $scope variable. Then we can see the output. Given bellow the “index.cshtml” file code:
@{
ViewBag.Title = "Home Page";
}
<div class="jumbotron" ng-controller="TestMy">
<table class="table table-bordered">
</table>
<ul class="pager">
<li>
<a href="#" ng-click="GetPageData()"><i class="glyphicon glyphicon-backward"></i></a>
</li>
</ul>
</div>
<script src="~/Scripts/customJs/HomeIndexController.js"></script>
Step-5
Now Build and run the project.