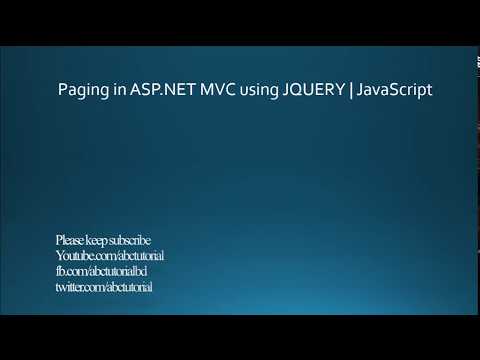
Download Project (Full Project)
Step-1
In this part i will show how to create paging by using Jquery Ajax. First open visual studio then creates a new ASP.Net MVC web application. Then select MVC and click ok. Visual studio create default project for you. Now create a folder name as Helper. This helper folder contain “PageData.cs” file. This file is responsible to get generic type data like “Data”, “CurrentPage”,” TotalPage” Property. Now add another class in same folder name is Pagination. This Pagination class method takes some page information which is generic type and also returns the data using the passing the parameter information. Now Connect the Ado.Net entity data model for get the data from database. Given bellow the PageData.cs code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace Jquery_Mvc_Paging.Helper
{
public class PagedData<T> where T:class
{
public IEnumerable<T> Data { get; set; }
public int TotalPages { get; set; }
public int CurrentPage { get; set; }
}
}
Step-2
This code is responsible to Pagination. Given bellow the Pagination.cs code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace Jquery_Mvc_Paging.Helper
{
public static class Pagination
{
public static PagedData<T> PagedResult<T>(this List<T> list, int PageNumber, int PageSize) where T : class
{
var result = new PagedData<T>();
result.Data = list.Skip(PageSize * (PageNumber - 1)).Take(PageSize).ToList();
result.TotalPages = Convert.ToInt32(Math.Ceiling((double)list.Count() / PageSize));
result.CurrentPage = PageNumber;
return result;
}
}
}
Step-3
Now controller are get data from database and this data pass the Paggination class. This Pagination class returns the page number with data. Also this controller returns the json data into JavaScript file. Given bellow the controller code:
using Jquery_Mvc_Paging.Helper;
using Jquery_Mvc_Paging.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace Jquery_Mvc_Paging.Controllers
{
public class HomeController : Controller
{
POSEntities context = new POSEntities();
public ActionResult Index()
{
return View();
}
public ActionResult GetPaggedData(int pageNumber = 1, int pageSize = 20)
{
List<Item> listData = context.Items.ToList();
var pagedData = Pagination.PagedResult(listData, pageNumber, pageSize);
return Json(pagedData, JsonRequestBehavior.AllowGet);
}
}
}
Step-4
Now “index.cshtml” file get the data by user input from server site and show the data in view. “JavaScript” file function call the c# method also pass the some value and also receive the json data and bind the Html tag using id and Display the view. Given bellow the “Index.cshtml” code:
@{
ViewBag.Title = "Home Page";
}
<br /><br />
<table class="table table-bordered">
<thead>
<tr>
<th>Id</th>
<th>Name</th>
</tr>
</thead>
<tbody id="tblData">
</tbody>
</table>
<div id="paged">
</div>
<script>
$(document).ready(function () {
//Initially load pagenumber=1
GetPageData(1);
});
function GetPageData(pageNum, pageSize) {
//After every trigger remove previous data and paging
$("#tblData").empty();
$("#paged").empty();
$.getJSON("/Home/GetPaggedData", { pageNumber: pageNum, pageSize: pageSize }, function (response) {
var rowData = "";
for (var i = 0; i < response.Data.length; i++) {
rowData = rowData + '<tr><td>' + response.Data[i].Id + '</td><td>' + response.Data[i].Name + '</td></tr>';
}
$("#tblData").append(rowData);
PaggingTemplate(response.TotalPages, response.CurrentPage);
});
}
//This is paging temlpate ,you should just copy paste
function PaggingTemplate(totalPage, currentPage) {
var template = "";
var TotalPages = totalPage;
var CurrentPage = currentPage;
var PageNumberArray = Array();
var countIncr = 1;
for (var i = currentPage; i <= totalPage; i++) {
PageNumberArray[0] = currentPage;
if (totalPage != currentPage && PageNumberArray[countIncr - 1] != totalPage) {
PageNumberArray[countIncr] = i + 1;
}
countIncr++;
};
PageNumberArray = PageNumberArray.slice(0, 5);
var FirstPage = 1;
var LastPage = totalPage;
if (totalPage != currentPage) {
var ForwardOne = currentPage + 1;
}
var BackwardOne = 1;
if (currentPage > 1) {
BackwardOne = currentPage - 1;
}
template = "<p>" + CurrentPage + " of " + TotalPages + " pages</p>"
template = template + '<ul class="pager">' +
'<li class="previous"><a href="#" onclick="GetPageData(' + FirstPage + ')"><i class="fa fa-fast-backward"></i> First</a></li>' +
'<li><select ng-model="pageSize" id="selectedId"><option value="20" selected>20</option><option value="50">50</option><option value="100">100</option><option value="150">150</option></select> </li>' +
'<li><a href="#" onclick="GetPageData(' + BackwardOne + ')"><i class="glyphicon glyphicon-backward"></i></a>';
var numberingLoop = "";
for (var i = 0; i < PageNumberArray.length; i++) {
numberingLoop = numberingLoop + '<a class="page-number active" onclick="GetPageData(' + PageNumberArray[i] + ')" href="#">' + PageNumberArray[i] + ' </a>'
}
template = template + numberingLoop + '<a href="#" onclick="GetPageData(' + ForwardOne + ')" ><i class="glyphicon glyphicon-forward"></i></a></li>' +
'<li class="next"><a href="#" onclick="GetPageData(' + LastPage + ')">Last <i class="fa fa-fast-forward"></i></a></li></ul>';
$("#paged").append(template);
$('#selectedId').change(function () {
GetPageData(1, $(this).val());
});
}
</script>
Step-5
Now build and run the project.