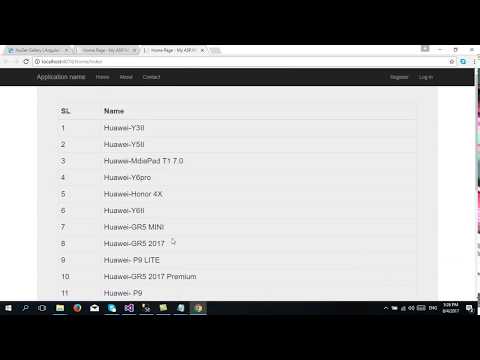
Download Project (Full Project)
Step-1
In this part i will show how to create paging in ASP.NET MVC using AngularJS. First open visual studio then runs our existing project where we are working. Now create a folder name as Helper. This helper folder contain “PageData.cs” file. This file is responsible to get generic type data like “Data”, “CurrentPage”, ” TotalPage” Property. Now add class in same folder name is Pagination. This class take some page information which is generic type and also return the data using the passing the parameter information. Now Connect the Ado.Net entity data model for get the data from database. Given bellow the “PageData.cs” code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace asp.net_mvc_pagging_angularjs.Helper
{
public class PagedData<T> where T:class
{
public IEnumerable<T> Data { get; set; }
public int TotalPages { get; set; }
public int CurrentPage { get; set; }
}
}
Step-2
This code is responsible to Pagination. Given bellow the Paggination.cs code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace asp.net_mvc_pagging_angularjs.Helper
{
public static class Paggination
{
public static PagedData<T> PagedResult<T>(this List<T> list, int PageNumber, int PageSize) where T : class
{
var result = new PagedData<T>();
result.Data = list.Skip(PageSize * (PageNumber - 1)).Take(PageSize).ToList();
result.TotalPages = Convert.ToInt32(Math.Ceiling((double)list.Count() / PageSize));
result.CurrentPage = PageNumber;
return result;
}
}
}
Step-3
Now controller are get data from database and this data pass the Pagination class. This Pagination class returns the page number with data. Also this controller returns the json data into JavaScript file. Given bellow the controller code:
using asp.net_mvc_pagging_angularjs.Helper;
using asp.net_mvc_pagging_angularjs.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace asp.net_mvc_pagging_angularjs.Controllers
{
public class HomeController : Controller
{
POSEntities context = new POSEntities();
public ActionResult Index()
{
return View();
}
public ActionResult GetPaggedData(int pageNumber=1,int pageSize=20)
{
List<Item> data = context.Items.ToList();
var paggedData = Paggination.PagedResult(data, pageNumber, pageSize);
return Json(paggedData, JsonRequestBehavior.AllowGet);
}
public ActionResult Contact()
{
ViewBag.Message = "Your contact page.";
return View();
}
}
}
Step-4
Now “HomeIndexController.js” file get the data by the user input from server site and show the data in view. HomeIndexController file function call the c# method also pass the some value and also receive the json data and bind the $scope veriable and Display the view. Given bellow the HomeIndexController.js code:
(function () {
app.controller('TestMy', function ($scope, $http) {
$scope.GetPageData = function (pageNum) {
$http.get('/Home/GetPaggedData', { params: { pageNumber: pageNum, pageSize: $scope.pageSize } }).then(function (response) {
$scope.Items = response.data.Data;
$scope.PaggingTemplate(response.data.TotalPages, response.data.CurrentPage);
});
};
var init = function () {
$scope.GetPageData(1);
};
init();
$scope.PaggingTemplate = function (totalPage, currentPage) {
$scope.showForwardBtn = true;
$scope.TotalPages = totalPage;
$scope.CurrentPage = currentPage;
$scope.PageNumberArray = Array();
var countIncr = 1;
for (var i = currentPage; i <= totalPage; i++) {
$scope.PageNumberArray[0] = currentPage;
if (totalPage != currentPage && $scope.PageNumberArray[countIncr - 1] != totalPage) {
$scope.PageNumberArray[countIncr] = i + 1;
}
countIncr++;
};
$scope.PageNumberArray = $scope.PageNumberArray.slice(0, 5);
$scope.FirstPage = 1;
$scope.LastPage = totalPage;
if (totalPage != currentPage) {
$scope.ForwardOne = currentPage + 1;
}
$scope.BackwardOne = currentPage - 1;
if (totalPage == currentPage) {
$scope.showForwardBtn = false;
}
};
});
}).call(angular);
Step-5
Then view is representing the data using html tag. In this view pass the data by ng-click and then the data post the server site and get the information by the angular. Then the angular store the data into $scope variable and show the data by using binding expiration in view. Given bellow the “index.cshtml” view code:
@{
ViewBag.Title = "Home Page";
}
<div class="jumbotron" ng-controller="TestMy">
<table class="table table-bordered">
<thead>
<tr>
<th>
Id
</th>
<th>
Name
</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="data in Items">
<td>{{data.Id}}</td>
<td>{{data.Name}}</td>
</tr>
</tbody>
</table>
<p>{{CurrentPage}} of {{TotalPages}} pages</p>
<ul class="pager">
<li class="previous"><a href="#" ng-click="GetPageData(FirstPage)"><i class="fa fa-fast-backward"></i> First</a></li>
<li>
<select ng-model="pageSize" ng-change="GetPageData(1)">
<option value="20" selected>20</option>
<option value="50">50</option>
<option value="100">100</option>
<option value="150">150</option>
</select>
</li>
<li>
<a href="#" ng-click="GetPageData(BackwardOne)"><i class="glyphicon glyphicon-backward"></i></a>
<a class="page-number active" ng-repeat="data in PageNumberArray" ng-click="GetPageData(data)" href="#">{{data}} </a>
<a href="#" ng-click="GetPageData(ForwardOne)" ng-show="showForwardBtn"><i class="glyphicon glyphicon-forward"></i></a>
</li>
<li class="next"><a href="#" ng-click="GetPageData(LastPage)">Last <i class="fa fa-fast-forward"></i></a></li>
</ul>
</div>
<script src="~/Scripts/customJs/HomeIndexController.js"></script>
Step-6
Now build and run the project.