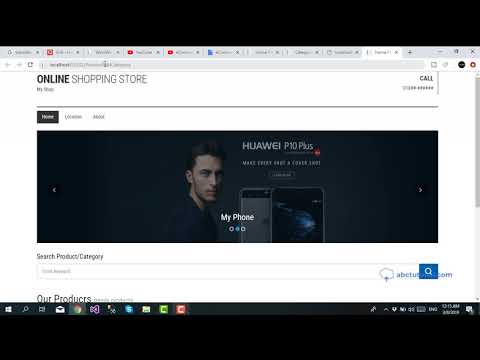
Download Project (Full Project)
Step-1
In this Part I will show how to add product and edit product. Open the project where we are working. First Add Admin controller under a action name is Product. This Product will create to show the product list. Add a method inside to Admin controller. This Product will create to show the product list. This product controller pass the product list in the view. Now view have edit button when click the edit button then the product object pass the controller and edit method are called from the Repository. Given bellow the Product Controller code:
public ActionResult Product()
{
return View(_unitOfWork.GetRepositoryInstance<Tbl_Product>().GetProduct());
}
Step-2
Add a View inside to View>Admin>Product.cshtml. Now this view recive the product table data and show the view. This view show Sr. No, Category Name, Action, Product, etc informetion. in this view have a add button this button are click to add new product. This view also have a edit button to edit each product informetion. Count is use to just show product number.
@model IEnumerable<OnlineShoppingStore.DAL.Tbl_Product>
@{
ViewBag.Title = "Product";
Layout = "~/Views/Shared/_AdminLayout.cshtml";
}
<div class="card mb-3">
<div class="card-header">
<i class="fas fa-table"></i>
Product
<a href="../Admin/ProductAdd" class="btn btn-info pull-right fa fa-plus">Add New</a>
</div>
<div class="card-body">
<div class="table-responsive">
<table class="table table-bordered" id="dataTable" width="100%" cellspacing="0">
<thead>
<tr>
<th>Sr. No.</th>
<th>Category Name</th>
<th>Action</th>
</tr>
</thead>
<tfoot>
<tr>
<th>Sr. No.</th>
<th>Product</th>
<th>Action</th>
</tr>
</tfoot>
<tbody>
@foreach (var item in Model)
{
int count = 1;
<tr>
<td>@count</td>
<td>@item.ProductName</td>
<td>
@Html.ActionLink("Edit", "ProductEdit", new { productId = item.ProductId })
</td>
</tr>
count++;
}
</tbody>
</table>
</div>
</div>
</div>
Step-3
Add a method inside Repository GenericRepository. GetProduct method are responsible to get product informetion from product table. when call the method from the controller then it pass the product table informetion.
public IEnumerable<Tbl_Entity> GetProduct()
{
return _dbSet.ToList();
}
Step-4
There is two mathod "ProductEdit()". One is http post and another is http get. http get are show the view and when it edit also pass the product informetion into view. And ProductEdit method http post receive the product informetion. and call the Repository under product table and call Update method to update the product informetion and save the database. Then redirect to the action product view.
Working Controller: AdminController
Create: ProductEdit
Code:
public ActionResult ProductEdit(int productId)
{
return View(_unitOfWork.GetRepositoryInstance<Tbl_Product>().GetFirstorDefault(productId));
}
[HttpPost]
public ActionResult ProductEdit(Tbl_Product tbl)
{
_unitOfWork.GetRepositoryInstance<Tbl_Product>().Update(tbl);
return RedirectToAction("Product");
}
Step-5
This is the product Add and Update view code. This view pass the single product informetion into the controller. BeginForm method pass the full-form informetion into the controlller when click save button.
@model OnlineShoppingStore.DAL.Tbl_Product
@{
ViewBag.Title = "ProductEdit";
Layout = "~/Views/Shared/_AdminLayout.cshtml";
}
<div class="container">
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div>
<h4>Product</h4>
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.ProductId, new { htmlAttributes = new { @class = "form-control" } })
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.ProductName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.ProductName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.ProductName, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.CategoryId, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.CategoryId, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.CategoryId, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.IsActive, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.IsActive, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.IsActive, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.IsDelete, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.IsDelete, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.IsDelete, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.CreatedDate, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.CreatedDate, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.CreatedDate, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.ModifiedDate, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.ModifiedDate, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.ModifiedDate, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.ProductImage, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.ProductImage, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.ProductImage, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.IsFeatured, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.IsFeatured, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.IsFeatured, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.Quantity, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Quantity, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Quantity, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
@Html.LabelFor(model => model.Price, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Price, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Price, "", new { @class = "text-danger" })
</div>
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Save" class="btn btn-default" />
</div>
</div>
</div>
</div>
}
</div>
Step-6
When the product informetion pass to controller from view then it receive ProductAdd controller. And call Repository under Add method to save the informetion into database. Then redirect to product view.
Working Controller: AdminController
Update: ProductAdd.cshtml
Code:
public ActionResult ProductAdd()
{
return View();
}
[HttpPost]
public ActionResult ProductAdd(Tbl_Product tbl)
{
_unitOfWork.GetRepositoryInstance<Tbl_Product>().Add(tbl);
return RedirectToAction("Product");
}
}
Step-7
Now run the project.