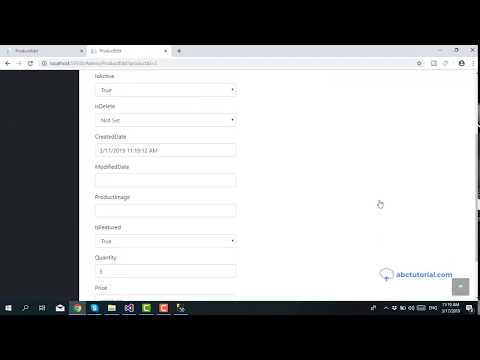
Download Project (Full Project)
Step-1
In this Part I will show how to Products Load and File upload. Open the project where we are working. First create GenericUnitOfWork of object. using _unitOfWork object under repository I get Category table from "GetAllRecords()" method. Push the all recode into list. Then AddCategory method return the list. And Categories method pass the all Categorie list into the view. UpdateCategorie method receive the id from the view and call the repository to get the data and convert to json then pass the json data into view. CatagoryEdit method get the id and pass the data into view using repository. Product controller pass the product list into the view. There is two product edit view one is get and another is post. Get method receive the id and find the data from table and pass the view and post method are receive the object and update the database. This product Edit controller also receive the image informetion and upload the iamge folder into our applicetion. Given bellow the Controller source code:
Admin Controller Source Code:
using Newtonsoft.Json;
using OnlineShoppingStore.DAL;
using OnlineShoppingStore.Models;
using OnlineShoppingStore.Repository;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace OnlineShoppingStore.Controllers
{
public class AdminController : Controller
{
// GET: Admin
public GenericUnitOfWork _unitOfWork = new GenericUnitOfWork();
public List<SelectListItem> GetCategory()
{
List<SelectListItem> list = new List<SelectListItem>();
var cat = _unitOfWork.GetRepositoryInstance<Tbl_Category>().GetAllRecords();
foreach (var item in cat)
{
list.Add(new SelectListItem { Value = item.CategoryId.ToString(), Text = item.CategoryName });
}
return list;
}
public ActionResult Dashboard()
{
return View();
}
public ActionResult Categories()
{
List<Tbl_Category> allcategories = _unitOfWork.GetRepositoryInstance<Tbl_Category>().GetAllRecordsIQueryable().Where(i => i.IsDelete == false).ToList();
return View(allcategories);
}
public ActionResult AddCategory()
{
return UpdateCategory(0);
}
public ActionResult UpdateCategory(int categoryId)
{
CategoryDetail cd;
if(categoryId != null)
{
cd = JsonConvert.DeserializeObject<CategoryDetail>(JsonConvert.SerializeObject(_unitOfWork.GetRepositoryInstance<Tbl_Category>().GetFirstorDefault(categoryId)));
}
else{
cd = new CategoryDetail();
}
return View("UpdateCategory", cd);
}
public ActionResult CategoryEdit(int catId)
{
return View(_unitOfWork.GetRepositoryInstance<Tbl_Category>().GetFirstorDefault(catId));
}
[HttpPost]
public ActionResult CategoryEdit(Tbl_Category tbl)
{
_unitOfWork.GetRepositoryInstance<Tbl_Category>().Update(tbl);
return RedirectToAction("Categories");
}
public ActionResult Product()
{
return View(_unitOfWork.GetRepositoryInstance<Tbl_Product>().GetProduct());
}
public ActionResult ProductEdit(int productId)
{
ViewBag.CategoryList = GetCategory();
return View(_unitOfWork.GetRepositoryInstance<Tbl_Product>().GetFirstorDefault(productId));
}
[HttpPost]
public ActionResult ProductEdit(Tbl_Product tbl, HttpPostedFileBase file)
{
string pic=null;
if (file != null)
{
pic = System.IO.Path.GetFileName(file.FileName);
string path = System.IO.Path.Combine(Server.MapPath("~/ProductImg/"), pic);
// file is uploaded
file.SaveAs(path);
}
tbl.ProductImage = file != null ? pic : tbl.ProductImage;
tbl.ModifiedDate = DateTime.Now;
_unitOfWork.GetRepositoryInstance<Tbl_Product>().Update(tbl);
return RedirectToAction("Product");
}
public ActionResult ProductAdd()
{
ViewBag.CategoryList = GetCategory();
return View();
}
[HttpPost]
public ActionResult ProductAdd(Tbl_Product tbl,HttpPostedFileBase file)
{
string pic=null;
if (file != null)
{
pic = System.IO.Path.GetFileName(file.FileName);
string path = System.IO.Path.Combine(Server.MapPath("~/ProductImg/"), pic);
// file is uploaded
file.SaveAs(path);
}
tbl.ProductImage = pic;
tbl.CreatedDate = DateTime.Now;
_unitOfWork.GetRepositoryInstance<Tbl_Product>().Add(tbl);
return RedirectToAction("Product");
}
}
}
Step-2
Product Add and Edit view. This view is pass Product informetion into controller using BeginForm method. All product informetion pass the using Tbl_product model. And when edit first data come form Tbl_product model and submited data pass the same model using int controller. Given bellow the view source code:
@model OnlineShoppingStore.DAL.Tbl_Product
@{
ViewBag.Title = "ProductAdd";
Layout = "~/Views/Shared/_AdminLayout.cshtml";
}
<div class="container">
@using (Html.BeginForm("ProductAdd", "Admin", FormMethod.Post, new { enctype = "multipart/form-data" }))
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h4>Product</h4>
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
<div class="form-group">
@Html.LabelFor(model => model.ProductName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.ProductName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.ProductName, "", new { @class = "text-danger" })
</div>
</div>
@{
List<SelectListItem> data=ViewBag.CategoryList;
}
<div class="form-group">
@Html.LabelFor(model => model.CategoryId, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.DropDownListFor(model => model.CategoryId, data, "---Select---", new { @class = "form-control" })
@Html.ValidationMessageFor(model => model.CategoryId, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.IsActive, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.CheckBox("IsActive")
@Html.ValidationMessageFor(model => model.IsActive, "", new { @class = "text-danger" })
</div>
</div>
@Html.HiddenFor(model => model.IsDelete)
<div class="form-group">
@Html.LabelFor(model => model.ProductImage, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
<input type="file" name="file" id="file" style="width: 100%;" />
@Html.ValidationMessageFor(model => model.ProductImage, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.IsFeatured, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.CheckBox("IsFeatured")
@Html.ValidationMessageFor(model => model.IsFeatured, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Quantity, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Quantity, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Quantity, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Price, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Price, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.Price, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Create" class="btn btn-default" />
</div>
</div>
</div>
}
</div>
Step-3
Product View show the all product list. This view have add new button to add the new product. This view show the Sr. No, Category Name, Action, Product etc also count is show for how many product in this list.
@model IEnumerable<OnlineShoppingStore.DAL.Tbl_Product>
@{
ViewBag.Title = "Product";
Layout = "~/Views/Shared/_AdminLayout.cshtml";
}
<div class="card mb-3">
<div class="card-header">
<i class="fas fa-table"></i>
Product
<a href="../Admin/ProductAdd" class="btn btn-info pull-right fa fa-plus">Add New</a>
</div>
<div class="card-body">
<div class="table-responsive">
<table class="table table-bordered" id="dataTable" width="100%" cellspacing="0">
<thead>
<tr>
<th>Sr. No.</th>
<th>Category Name</th>
<th>Action</th>
</tr>
</thead>
<tfoot>
<tr>
<th>Sr. No.</th>
<th>Product</th>
<th>Action</th>
</tr>
</tfoot>
<tbody>
@foreach (var item in Model)
{
int count = 1;
<tr>
<td>@count</td>
<td>@item.ProductName</td>
<td>
@Html.ActionLink("Edit", "ProductEdit", new { productId = item.ProductId })
</td>
</tr>
count++;
}
</tbody>
</table>
</div>
</div>
</div>
Step-4
Category View show the all category list. This view have add new button to add the new category. This view show the Sr. No, Category Name, Action etc also count is show for how many product in this list.
@model List<OnlineShoppingStore.DAL.Tbl_Category>
@{
ViewBag.Title = "Categories";
Layout = "~/Views/Shared/_AdminLayout.cshtml";
}
<div class="container-fluid">
<!-- Breadcrumbs-->
<ol class="breadcrumb">
<li class="breadcrumb-item">
<a href="#">Dashboard</a>
</li>
<li class="breadcrumb-item active">Categories</li>
</ol>
<!-- DataTables Example -->
<div class="card mb-3">
<div class="card-header">
<i class="fas fa-table"></i>
Category Details
<a href="../Admin/AddCategory" class="btn btn-info pull-right fa fa-plus">Add New</a>
</div>
<div class="card-body">
<div class="table-responsive">
<table class="table table-bordered" id="dataTable" width="100%" cellspacing="0">
<thead>
<tr>
<th>Sr. No.</th>
<th>Category Name</th>
<th>Action</th>
</tr>
</thead>
<tfoot>
<tr>
<th>Sr. No.</th>
<th>Category Name</th>
<th>Action</th>
</tr>
</tfoot>
<tbody>
@foreach (var item in Model)
{
<tr>
<td>@(Model.IndexOf(item)+1)</td>
<td>@item.CategoryName</td>
<td>
@Html.ActionLink("Edit", "CategoryEdit", new { catId = item.CategoryId })
</td>
</tr>
}
</tbody>
</table>
</div>
</div>
</div>
</div>
Step-5
This is Category Add and Edit view when the Tbl_Category method is get null then it is add method and when it pass the object then edit method. BeginForm is responsible for post the all fields by using save button click pass object into the controlle:
@model OnlineShoppingStore.DAL.Tbl_Category
@{
ViewBag.Title = "CategoryEdit";
Layout = "~/Views/Shared/_AdminLayout.cshtml";
}
<div class="container">
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h4>Category</h4>
<hr />
@Html.ValidationSummary(true, "", new { @class = "text-danger" })
@Html.HiddenFor(model => model.CategoryId)
<div class="form-group">
@Html.LabelFor(model => model.CategoryName, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.CategoryName, new { htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.CategoryName, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.IsActive, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.CheckBox("IsActive")
@Html.ValidationMessageFor(model => model.IsActive, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.IsDelete, htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.CheckBox("IsDelete")
@Html.ValidationMessageFor(model => model.IsDelete, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Save" class="btn btn-default" />
</div>
</div>
</div>
}
</div>
Step-6
Run the project.