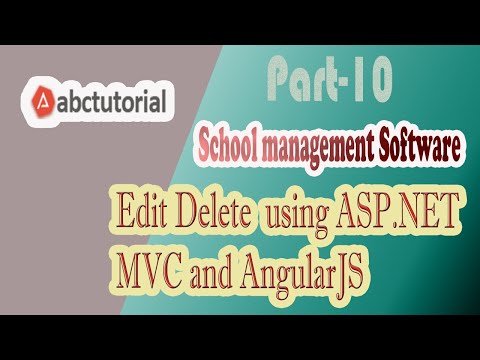
Part-04: Bootstrap Admin Dashboard Template setup in School Management Software
Part 5: How to use AngularJS in ASP.NET MVC
Part-6: CRUD Operation Insert Data using AngularJS in ASP.NET MVC
Part-7: CRUD Operation & Load Data using AngularJS in ASP.NET MVC
Part-08: CRUD Operation Edit, Delete Data using Angularjs in ASP.NET MVC
Part-3: Create Database and Table in sql server for school management system
Part-09: Insert Section data using ASP.NET MVC AngularJs
Part-10: Edit Update and Delete Section data using Angular js | ASP.NET MVC | Jquery
Part-11: Cascading Dropdownlist Section Batch selection in asp.net MVC JQUERY AngularJS
Part-12: Insert & Delete course information for school management software using ASP.NET MVC Javascript Angularjs
Part-13: Create Update course info in ASP.NET MVC AngularJs JQUERY Javascript
Part-14: Insert data and Page design bootstrap using ASP.NET MVC JQUERY AngularJS
Part-15: Insert & Get data using store procedure in SQL Server ASP.NET MVC AngularJS JQUERY
Part-16: Load student list,bootstrap and Inactive using ASP.NET MVC AngularJS Jquery
Part-17: User authentication using Store procedure Javascript AngularJS JQUERY ASP.NET MVC
Part-18: User authentication, authorization and login using ASP.NET MVC AngularJS Javascript JQUERY
Part-19: User Registration & Insert semester Info using AngularJS in ASP.NET MVC
Part-20: Load semester info & Student course offer page design using ASP.NET MVC JQUERY Angularjs
Part-21: Course Offer Entry Using Jquery Multiple Data Save (Part-1) using ASP.NET MVC AngularJS
SMS-22: Student Course Offer Entry happens Using Jquery Multiple Data Save List view dropdownlist Load using Angular js in ASP.NET MVC
SMS-23: Student course offer list semester search using ASP.NET MVC AngularJS JQUERY
SMS-24: Student Marks Entry page in table column input marks entry using Jquery & Angular js in ASP.NET MVC
SMS-25: Student Course Mark multiple data save using jquery with Stored Procedure & Angular js in ASP.NET MVC
SMS-26: Student Marks list show by search student name and trimester Jquery & Angular js in ASP.NET MVC
SMS-27: Student profile create and browse profile using Store procedure AngularJS Jquery ASP.NET
SMS-28: Student Result show by search trimester and myasp server registration and login using Jquery & Angular js in ASP.NET MVC
In this tutorial I will show to Load Data, Edit/Update and Delete Section data using Angular js in ASP.NET MVC. If you follow then will learn update, delete and load data using asp.net mvc
Steps:
Step-1:
- Add LoadData() JsonResult in SectionController.cs.
- Go to Solution Explorer > Controllers Folder> SectionController > in this controller with writing the below code.
public JsonResult LoadData()
{
DataSet ds = aDal.LoadAllDataDAL();
List<SectionDAO> lists = new List<SectionDAO>();
foreach (DataRow dr in ds.Tables[0].Rows)
{
lists.Add(new SectionDAO
{
SectionId = Convert.ToInt32(dr["SectionId"]),
SL = (dr["SL"].ToString()),
SectionName = (dr["SectionName"].ToString()),
BatchName = (dr["BatchName"].ToString())
});
}
return Json(lists, JsonRequestBehavior.AllowGet);
}
Step-2:
- Add LoadAllDataDAL() in SectionDAL.cs Class .
- Go to Solution Explorer > DAL Folder> SectionDAL > in this controller with writing the below code.
public DataSet LoadAllDataDAL()
{
SqlCommand com = new SqlCommand("sp_LoadAllData_Section", conn);
com.CommandType = CommandType.StoredProcedure;
SqlDataAdapter da = new SqlDataAdapter(com);
DataSet dss = new DataSet();
da.Fill(dss);
return dss;
}
Step-3:
- Create SectionControllerJS.js file.
- Go to Solution Explorer > Scripts Folder.> AngularController Folder> Create ‘SectionControllerJS.js’> for Batch Name Load with writing the below code.
$http.get("/Section/ddlLoadBatch").then(function (d) {
$scope.Batch = d.data;
}, function (error) {
alert("Faild");
});
Step-4:
In SectionController.cs class add ddlLoadBatch() JsonResult for Load Batch Name.
Go to Solution Explorer > Controllers Folder > SectionController.cs in this controller with writing the below code.
public JsonResult ddlLoadBatch()
{
DataSet ds = aDal.BatchDDLLoadDAL();
List<BatchDAO> lists = new List<BatchDAO>();
foreach (DataRow dr in ds.Tables[0].Rows)
{
lists.Add(new BatchDAO
{
BatchId = Convert.ToInt32(dr["BatchId"]),
BatchName = (dr["BatchName"].ToString())
});
}
return Json(lists, JsonRequestBehavior.AllowGet);
}
Step-5:
- Create store Procedure for Load Section Info.
- Go to SQL Server 2014 > dbStudentMangeSystem database> Programmability> stored procedures> Select New> stored procedure>Create sp_LoadAllData_Section with writing the below code.
create proc [dbo].[sp_LoadAllData_Section]
as
begin
select ROW_NUMBER() over (order by Sec.SectionId) as SL, ba.BatchName, * from tblSection as Sec
left join tblBatch ba on ba.BatchId=Sec.BatchId
end
Step-6:
- Add Load Data function in SectionControllerJS.js file.
- Go to Solution Explorer > Scripts Folder.> AngularController Folder> ‘SectionControllerJS.js’> for Load Section Information with writing the below code.
$http.get("/Section/LoadData").then(function (d) {
$scope.Section = d.data;
}, function (error) {
alert("Faild");
});
Step-7:
- Add Codes in SectionList.cshtml Page.
- Go to Solution Explorer > Views Folder > Section Folder> SectionList.cshtml in this page with writing the below code.
<div ng-app="ABCApp" ng-controller="SectionController">
<div class="content-wrapper">
<!-- Content Header (Page header) -->
<div class="content-header">
<div class="container-fluid">
<div class="row mb-2">
<div class="col-sm-6">
<h1 class="m-0 text-dark">Section</h1>
</div><!-- /.col -->
<div class="col-sm-6">
<ol class="breadcrumb float-sm-right">
<li class="breadcrumb-item"><a href="../Section/SectionEntry">Add New Info</a></li>
</ol>
</div><!-- /.col -->
</div><!-- /.row -->
</div><!-- /.container-fluid -->
</div>
<!-- /.content-header -->
<!-- Main content -->
<section class="content">
<div class="container-fluid">
<div class="row">
<div class="col-md-12">
<table class="table table-responsive">
<tr>
<td>SL#</td>
<td>Batch Name</td>
<td>Section Name</td>
<td>Edit</td>
<td>Delete</td>
</tr>
<tr ng-repeat="e in Section" ng-class-even="'even'" ng-class-odd="'odd'">
<td>{{e.SL}}</td>
<td>{{e.BatchName}}</td>
<td>{{e.SectionName}}</td>
<td>
<a href="/Section/SectionEntry?MasterId={{e.SectionId}}" class="btn btn-warning">Edit</a>
</td>
<td><a ng-click="DeleteMAsterData(e.SectionId)" class="btn btn-danger">Delete</a> </td>
</tr>
</table>
</div>
</div>
</div>
</section>
</div>
</div>
<script src="~/Scripts/angular.min.js"></script>
<script src="~/Scripts/AngularController/SectionControllerJS.js"></script>
Step-8:
- In SectionController.cs class add GetMAsterDataByID() JsonResult for Get One Record.
- Go to Solution Explorer > Controllers Folder > SectionController.cs in this controller with writing the below code.
public JsonResult GetMAsterDataByID(int MasterId)
{
DataSet ds = aDal.LoadDataByMasterIDDAL(MasterId);
List<SectionDAO> lists = new List<SectionDAO>();
foreach (DataRow dr in ds.Tables[0].Rows)
{
lists.Add(new SectionDAO
{
SectionId = Convert.ToInt32(dr["SectionId"]),
SectionName = Convert.ToString(dr["SectionName"]),
BatchId = Convert.ToInt32(dr["BatchId"]),
});
}
return Json(lists, JsonRequestBehavior.AllowGet);
}
Step-9:
- Add Load Data function in SectionControllerJS.js file.
- Go to Solution Explorer > Scripts Folder.> AngularController Folder> ‘SectionControllerJS.js’> for GetOneRecord with writing the below code.
$scope.GetOneRecord = function (MasterId) {
if (MasterId != "0") {
$http.get("/Section/GetMAsterDataByID?MasterId=" + MasterId).then(function (d) {
$scope.CourseDAO = d.data[0];
}, function (error) {
alert("Faild");
});
}
};
Step-10:
- In SectionController.cs class add Update_Info() JsonResult for update section information.
- Go to Solution Explorer > Controllers Folder > SectionController.cs in this controller with writing the below code.
public JsonResult Update_Info(SectionDAO aDao)
{
string Mes = "";
try
{
aDal.UpdateInfoDAL(aDao);
Mes = "Operation Successful!!";
}
catch (Exception e)
{
Mes = "Operation Faild!!";
}
return Json(Mes, JsonRequestBehavior.AllowGet);
}
Step-11:
- Add UpdateInfoDAL() in SectionDAL.cs Class .
- Go to Solution Explorer > DAL Folder> SectionDAL > in this controller with writing the below code.
public void UpdateInfoDAL(SectionDAO aDao)
{
SqlCommand com = new SqlCommand("Update_SectionByMasterId", conn);
com.CommandType = CommandType.StoredProcedure;
com.Parameters.AddWithValue("@SectionId", aDao.SectionId);
com.Parameters.AddWithValue("@SectionName", aDao.SectionName);
com.Parameters.AddWithValue("@BatchId", aDao.BatchId);
conn.Open();
com.ExecuteNonQuery();
conn.Close();
}
Step-12:
- Create store Procedure for Load Section Info.
- Go to SQL Server 2014 > dbStudentMangeSystem database> Programmability> stored procedures> Select New> stored procedure>Create Update_SectionByMasterId with writing the below code.
create proc [dbo].[Update_SectionByMasterId]
@SectionId int,
@BatchId int,
@SectionName nvarchar(500)
as
begin
update tblSection set BatchId=@BatchId,SectionName= @SectionName where SectionId=@SectionId
end
Step-13:
- Add SaveData function in SectionControllerJS.js file.
- Go to Solution Explorer > Scripts Folder.> AngularController Folder> ‘SectionControllerJS.js’> for Update Section information with writing the below highlight code.
$scope.SaveData = function() {
if ($scope.btnSaveText == "Save") {
$scope.btnSaveText = "Saving.....";
$http({
method: 'POST',
url: '/Section/Save_Info',
data: $scope.SectionDAO
}).success(function(a) {
$scope.btnSaveText = "Save";
$scope.SectionDAO = null;
alert(a);
}).error(function() {
alert("Faild");
});
} else {
$scope.btnSaveText = "Updating.....";
$http({
method: 'POST',
url: '/Section/Update_Info',
data: $scope.SectionDAO
}).success(function (a) {
$scope.btnSaveText = "Save";
$scope.SectionDAO = null;
alert(a);
}).error(function () {
alert("Faild");
});
}
};
Step-14:
- In SectionController.cs class add ReomveDataByMAsterId() JsonResult for update section information.
- Go to Solution Explorer > Controllers Folder > SectionController.cs in this controller with writing the below code.
public JsonResult ReomveDataByMAsterId(int SectionId)
{
string result = string.Empty;
try
{
aDal.DeleteInfoDAL(SectionId);
result = "Operation Deleted";
}
catch (Exception)
{
result = "Operation Faild";
//throw;
}
return Json(result, JsonRequestBehavior.AllowGet);
}
Step-15:
- Add DeleteInfoDAL() in SectionDAL.cs Class .
- Go to Solution Explorer > DAL Folder> SectionDAL > in this controller with writing the below code.
public void DeleteInfoDAL(int SectionId)
{
SqlCommand com = new SqlCommand("Delete_SectionByMasterId", conn);
com.CommandType = CommandType.StoredProcedure;
com.Parameters.AddWithValue("@SectionId", SectionId);
conn.Open();
com.ExecuteNonQuery();
conn.Close();
}
Step-16:
- Create store Procedure for Delete Section Info.
- Go to SQL Server 2014 > dbStudentMangeSystem database> Programmability> stored procedures> Select New> stored procedure>Create Delete_SectionByMasterId with writing the below code.
create proc [dbo].[Delete_SectionByMasterId]
@SectionId int
as
begin
delete from tblSection where SectionId=@SectionId
end
Step-12:
- Add SaveData function in SectionControllerJS.js file.
- Go to Solution Explorer > Scripts Folder.> AngularController Folder> ‘SectionControllerJS.js’> for Delete Section information with writing the below code.
$scope.DeleteMAsterData = function (SectionId) {
$http.get("/Section/ReomveDataByMAsterId?SectionId=" + SectionId).then(function (d) {
alert(d.data);
$http.get("/Section/LoadData").then(function (d) {
$scope.Section = d.data;
}, function (error) {
alert("Faild");
});
},
function (error) {
alert("Faild");
}
);
};
Step-13: Run Application.