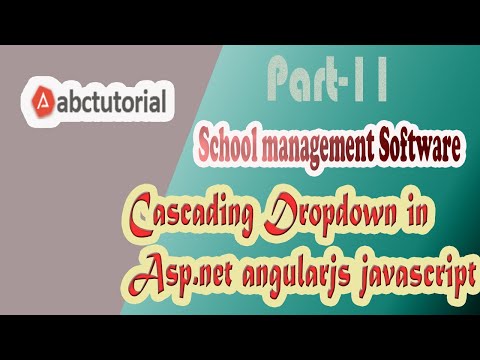
Part-04: Bootstrap Admin Dashboard Template setup in School Management Software
Part 5: How to use AngularJS in ASP.NET MVC
Part-6: CRUD Operation Insert Data using AngularJS in ASP.NET MVC
Part-7: CRUD Operation & Load Data using AngularJS in ASP.NET MVC
Part-08: CRUD Operation Edit, Delete Data using Angularjs in ASP.NET MVC
Part-3: Create Database and Table in sql server for school management system
Part-09: Insert Section data using ASP.NET MVC AngularJs
Part-10: Edit Update and Delete Section data using Angular js | ASP.NET MVC | Jquery
Part-11: Cascading Dropdownlist Section Batch selection in asp.net MVC JQUERY AngularJS
Part-12: Insert & Delete course information for school management software using ASP.NET MVC Javascript Angularjs
Part-13: Create Update course info in ASP.NET MVC AngularJs JQUERY Javascript
Part-14: Insert data and Page design bootstrap using ASP.NET MVC JQUERY AngularJS
Part-15: Insert & Get data using store procedure in SQL Server ASP.NET MVC AngularJS JQUERY
Part-16: Load student list,bootstrap and Inactive using ASP.NET MVC AngularJS Jquery
Part-17: User authentication using Store procedure Javascript AngularJS JQUERY ASP.NET MVC
Part-18: User authentication, authorization and login using ASP.NET MVC AngularJS Javascript JQUERY
Part-19: User Registration & Insert semester Info using AngularJS in ASP.NET MVC
Part-20: Load semester info & Student course offer page design using ASP.NET MVC JQUERY Angularjs
Part-21: Course Offer Entry Using Jquery Multiple Data Save (Part-1) using ASP.NET MVC AngularJS
SMS-22: Student Course Offer Entry happens Using Jquery Multiple Data Save List view dropdownlist Load using Angular js in ASP.NET MVC
SMS-23: Student course offer list semester search using ASP.NET MVC AngularJS JQUERY
SMS-24: Student Marks Entry page in table column input marks entry using Jquery & Angular js in ASP.NET MVC
SMS-25: Student Course Mark multiple data save using jquery with Stored Procedure & Angular js in ASP.NET MVC
SMS-26: Student Marks list show by search student name and trimester Jquery & Angular js in ASP.NET MVC
SMS-27: Student profile create and browse profile using Store procedure AngularJS Jquery ASP.NET
SMS-28: Student Result show by search trimester and myasp server registration and login using Jquery & Angular js in ASP.NET MVC
This tutorial will help you to learn Cascading Dropdownlist Batch and Section Using ASP.NET MVC JQUERY AngularJS Javascript.
Steps:
Step-1:
- Add Controller CourseController.cs.
- Go to Solution Explorer > Controllers Folder> Add > Controller> Select MVC 5 Controller-Empty> Click ‘Add’ button> Give Controller Name ’ CourseController’ > in this controller with writing the below code.
public ActionResult CourseListView()
{
return View();
}
- In the CourseListView() Action Result Mouse right button Select Add View > Click Add Button> CourseListView.cshtml page has been created.
- Add Another Action result CourseEntry() with writing the below code.
public ActionResult CourseEntry()
{
return View();
}
- In the CourseEntry() Action Result Mouse right button Select Add View > Click Add Button> CourseEntry.cshtml page has been created.
Step-2:
- Modify CourseEntry.cshtml page with writing the below code.
<div ng-app="ABCApp" ng-controller="CourseController" data-ng-init="GetOneRecord(@Convert.ToInt32(Request.QueryString["MasterId"]))">
<div class="content-wrapper">
<!-- Content Header (Page header) -->
<div class="content-header">
<div class="container-fluid">
<div class="row mb-2">
<div class="col-sm-6">
<h1 class="m-0 text-dark">Course Entry </h1>
</div><!-- /.col -->
<div class="col-sm-6">
<ol class="breadcrumb float-sm-right">
<li class="breadcrumb-item"><a href="../Course/CourseListView">List View</a></li>
</ol>
</div><!-- /.col -->
</div><!-- /.row -->
</div><!-- /.container-fluid -->
</div>
<!-- /.content-header -->
<!-- Main content -->
<section class="content">
<div class="container-fluid">
<div class="row">
<div class="col-md-3">
<div class="form-group">
<label>Batch Name</label>
<select class="form-control" ng-model="CourseDAO.BatchId" ng-change="LoadSectionByBatchID(CourseDAO.BatchId)">
<option ng-repeat="e in Batch" value="{{e.BatchId}}">{{e.BatchName}}</option>
</select>
</div>
</div>
</div>
<div class="row">
<div class="col-md-3">
<div class="form-group">
<label>Section Name</label>
<select class="form-control" ng-model="CourseDAO.SectionId">
<option ng-repeat="e in Section" value="{{e.SectionId}}">{{e.SectionName}}</option>
</select>
</div>
</div>
</div>
<div class="row">
<div class="col-md-3">
<div class="form-group">
<label>Course Name</label>
<input type="text" ng-model="CourseDAO.CourseName" class="form-control" />
</div>
</div>
</div>
<div class="row">
<div class="col-md-3">
<input type="button" ng-click="SaveData()" class="btn btn-primary" value="{{btnSaveTextCo}}" />
</div>
</div>
</div>
</section>
</div>
</div>
<script src="~/Scripts/angular.min.js"></script>
<script src="~/Scripts/AngularController/CourseControllerJS.js"></script>
Step-3:
- Add ddlLoadBatch() JsonResult for Batch Name in CourseController.cs.
- Go to Solution Explorer > Controllers Folder> CourseController> in this controller with writing the below code.
public JsonResult ddlLoadBatch()
{
DataSet ds = aDal.BatchDDLLoadDAL();
List<BatchDAO> lists = new List<BatchDAO>();
foreach (DataRow dr in ds.Tables[0].Rows)
{
lists.Add(new BatchDAO
{
BatchId = Convert.ToInt32(dr["BatchId"]),
BatchName = (dr["BatchName"].ToString())
});
}
return Json(lists, JsonRequestBehavior.AllowGet);
}
Step-4:
- Create CourseDAL.cs class.
- Go to Solution Explorer > DAL Folder > CourseDAL.cs Class with writing the below code.
SqlConnection conn = new SqlConnection(ConfigurationManager.ConnectionStrings["MyConStr"].ConnectionString);
public DataSet BatchDDLLoadDAL()
{
SqlCommand com = new SqlCommand("sp_BatchDDLLoad", conn);
com.CommandType = CommandType.StoredProcedure;
SqlDataAdapter da = new SqlDataAdapter(com);
DataSet dss = new DataSet();
da.Fill(dss);
return dss;
}
Step-5:
- Create CourseControllerJS.js file.
- Go to Solution Explorer > Scripts Folder.> AngularController Folder> Create ‘CourseControllerJS.js> for Batch Name Load with writing the below code.
$http.get("/Course/ddlLoadBatch").then(function (d) {
$scope.Batch = d.data;
}, function (error) {
alert("Faild");
});
});
- When you select Batch Name dropdownlist then Section name will be loaded from the database against Batch Name.
Step-6:
- Add ddlLoadSectonbyBatchID() JsonResult for section Name in CourseController.cs.
- Go to Solution Explorer > Controllers Folder> CourseController> in this controller with writing the below code.
public JsonResult ddlLoadSectonbyBatchID(int BatchId)
{
DataSet ds = aDal.SectionByBathIdDDLLoadDAL(BatchId);
List<SectionDAO> lists = new List<SectionDAO>();
foreach (DataRow dr in ds.Tables[0].Rows)
{
lists.Add(new SectionDAO
{
SectionId = Convert.ToInt32(dr["SectionId"]),
SectionName = (dr["SectionName"].ToString())
});
}
return Json(lists, JsonRequestBehavior.AllowGet);
}
Step-7:
- Create SectionDAO.cs Class.
- Go to Solution Explorer > DAO Folder> create SectionDAO> in this class with writing the below code.
public class SectionDAO
{
public int SectionId { get; set; }
public string SL { get; set; }
public string SectionName { get; set; }
public int BatchId { get; set; }
public string BatchName { get; set; }
}
Step-8:
- Add SectionByBathIdDDLLoadDAL() in CourseDAL.cs class.
- Go to Solution Explorer > DAL Folder > CourseDAL.cs Class with writing the below code.
public DataSet SectionByBathIdDDLLoadDAL(int BatchId)
{
SqlCommand com = new SqlCommand("sp_SectionByBatchIdDDLLoad", conn);
com.CommandType = CommandType.StoredProcedure;
com.Parameters.AddWithValue("@BatchId", BatchId);
SqlDataAdapter da = new SqlDataAdapter(com);
DataSet dss = new DataSet();
da.Fill(dss);
return dss;
}
Step-9:
- Create store Procedure for Section Name.
- Go to SQL Server 2014 > dbStudentMangeSystem database> Programmability> stored procedures> Select New> stored procedure>Create sp_SectionByBatchIdDDLLoad with writing the below code.
create proc [dbo].[sp_SectionByBatchIdDDLLoad]
@BatchId int
as
begin
select SectionId,SectionName from tblSection where BatchId=@BatchId order by SectionName
end
Step-10:
- In CourseControllerJS.js add LoadSectionByBatchID Function.
- Go to Solution Explorer > Scripts Folder > CourseControllerJS.js with writing the below code.
$scope.LoadSectionByBatchID = function(BatchId) {
$http.get("/Course/ddlLoadSectonbyBatchID?BatchId=" + BatchId).then(function(d) {
$scope.Section = d.data;
}, function(error) {
alert("Faild");
});
};
Step-11: Run Application.