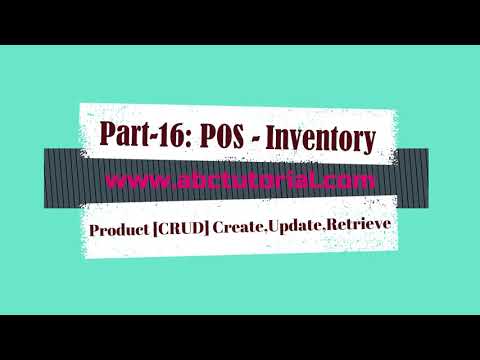
Post your any code related problem to www.abctutorial.com
Follow the previous video and articles to complete the POS tutorials
Step-1
Here we are going to create update retrieve of Product which will use in entire POS & Inventory Project. Actually, this is the CRUD operation which I showed you in several videos so that why it’s the repetitive video of CRUD. If you follow this article, then you will have the clear understanding of the CRUD operation.
- Please add a method under HomeController
[AuthorizationFilter]
public ActionResult Product()
{
return View();
}
- Add below code into Product.cshtml.
@{
ViewBag.Title = "Product";
}
<div class="col-xl-12 col-md-12 mb-12">
<div class="card border-left-primary shadow h-100 py-2">
<div class="card-body">
<div class="row no-gutters align-items-center">
<div class="col mr-2">
<form class="user">
<div class="form-group col-md-6">
<div class="text-xs font-weight-bold text-primary text-uppercase mb-1">Create Product</div>
</div>
<input type="hidden" id="productId" />
<div class="form-group col-md-6">
<input type="text" class="form-control form-control-user" id="productName" aria-describedby="emailHelp" placeholder="Product">
</div>
<div class="form-group col-md-6">
<select class="form-control" id="categoryId"></select>
</div>
<div class="form-group col-md-6">
<select class="form-control" id="status">
<option>--Select--</option>
<option value="1">Active</option>
<option value="0">Inactive</option>
</select>
</div>
<div class="form-group col-md-6">
<a href="#" onclick="SaveProduct()" class="btn btn-primary btn-sm">
Save
</a>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
<br />
<div class="col-xl-12 col-md-12 mb-12">
<div class="card border-left-primary shadow h-100 py-2">
<div class="card-body">
<div class="row no-gutters align-items-center">
<div class="col mr-2">
<div class="form-group col-md-6">
<div class="text-xs font-weight-bold text-primary text-uppercase mb-1">Product List</div>
</div>
<table class="table table-bordered" id="dataTable" width="100%" cellspacing="0">
<thead>
<tr>
<th>Name</th>
<th>Category</th>
<th>Status</th>
<th>
Action
</th>
</tr>
</thead>
<tbody id="trDiv"></tbody>
</table>
</div>
</div>
</div>
</div>
</div>
Step-2
The main part of this Product CRUD is saving, updating, retrieving data so here I create and update data using one single method SaveProduct
- Please go to HomeController
- Add this below method inside the HomeController
[HttpPost]
public JsonResult SaveProduct(Product product)
{
POS_TutorialEntities db = new POS_TutorialEntities();
bool isSuccess = true;
if (product.ProductId > 0)
{
db.Entry(product).State = EntityState.Modified;
}
else
{
db.Products.Add(product);
}
try
{
db.SaveChanges();
}
catch (Exception)
{
isSuccess = false;
}
return Json(isSuccess, JsonRequestBehavior.AllowGet);
}
Step-3
As a part of CRUD, to updating data we must show list of data to select for updating. Please follow below steps to
- Please go to HomeController.
- Please write this below code to show list of Product.
[HttpGet]
public JsonResult GetAllProduct()
{
POS_TutorialEntities db = new POS_TutorialEntities();
var dataList = db.Products.Include("Category").ToList();
var modefiedData = dataList.Select(x => new
{
ProductId = x.ProductId,
CategoryId = x.CategoryId,
Name=x.Name,
Status=x.Status,
CategoryName=x.Category.Name
}).ToList();
return Json(modefiedData, JsonRequestBehavior.AllowGet);
}
Step-4
As we are implementing CRUD operation here, we used JQUERY AJAX JSON so we will add few JavaScript code which will save, update, retrieve data.
- Please go to Product.cshtml view.
- Please add this below JS code in below of all HTML code.
<script>
$(function () {
GetAllProduct();
GetAllGetegory();
});
function SaveProduct() {
var product = new Object();
product.ProductId = $("#productId").val();
product.CategoryId = $("#categoryId").val();
product.Name = $("#productName").val();
product.Status = $("#status").val();
var data = JSON.stringify({
product: product
});
return $.ajax({
contentType: 'application/json; charset=utf-8',
dataType: 'json',
type: 'POST',
url: "/Home/SaveProduct",
data: data,
success: function (result) {
if (result == true) {
GetAllProduct();
Reset();
alert("Save Success!");
}
else {
alert("Save failed!");
}
},
error: function () {
alert("Error!")
}
});
}
function GetAllProduct() {
$.ajax({
contentType: 'application/json; charset=utf-8',
dataType: 'json',
type: 'Get',
url: "/Home/GetAllProduct",
success: function (data) {
$("#trDiv").html('');
var tdContent = '';
for(var i = 0; i < data.length; i++)
{
var statuName = "";
if (data[i].Status == 1) {
statusName = "Active";
}
else {
statusName = "Inactive";
}
tdContent += '<tr><td>' + data[i].Name + '</td>' + '<td>' + data[i].CategoryName + '</td>' + '<td>' + statusName + '</td>' + '<td><a href="#" onclick="Edit(' + data[i].ProductId + "," + data[i].CategoryId + ",'" + data[i].Name + "'," + data[i].Status + ');">Edit</a></td></tr>'; // if Name is property of your Person object
}
$("#trDiv").append(tdContent);
},
error: function () {
alert("Error!")
}
});
}
function GetAllGetegory() {
$.ajax({
contentType: 'application/json; charset=utf-8',
dataType: 'json',
type: 'Get',
url: "/Home/GetAllGetegory",
success: function (data) {
$("#categoryId").html('');
var tdContent = '';
for (var i = 0; i < data.length; i++) {
tdContent += '<option value="'+data[i].CategoryId+'">' + data[i].Name +'</option>'; // if Name is property of your Person object
}
$("#categoryId").append(tdContent);
},
error: function () {
alert("Error!")
}
});
}
function Edit(productId, catId, name, status) {
$("#productId").val(productId);
$("#categoryId").val(catId);
$("#productName").val(name);
$("#status").val(status);
}
function Reset() {
$("#productId").val(null);
$("#categoryId").val(null);
$("#productName").val(null);
$("#status").val(null);
}
</script>
- Now run the project.
- Then save, update, retrieve data.