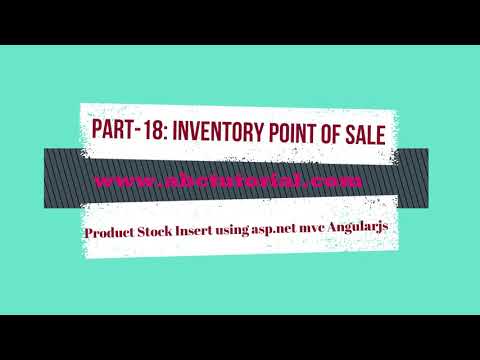
Post your any code related problem to www.abctutorial.com
Follow the previous video and articles to complete the POS tutorials
Step-1
Here we are going to create Insert operation of Product Stock Entry which will use in entire POS & Inventory Project. Actually this is the CRUD operation which I showed you in several. If you follow this article then you would be able to do the CRUD operation as well.
- Please configure AngularJS first in your project by following this link
- Please add a method under HomeController
[AuthorizationFilter]
public ActionResult ProductStock()
{
return View();
}
- Add below code into ProductStock.cshtml.
@{
ViewBag.Title = "ProductStock";
}
<div ng-controller="ProductStock">
<div class="col-xl-12 col-md-12 mb-12">
<div class="card border-left-primary shadow h-100 py-2">
<div class="card-body">
<div class="row no-gutters align-items-center">
<div class="col mr-2">
<form class="user">
<div class="form-group col-md-6">
<div class="text-xs font-weight-bold text-primary text-uppercase mb-1">Create Product</div>
</div>
<div class="form-group col-md-6">
<select class="form-control" ng-model="ProductStock.ProductId">
<option ng-repeat="item in ProductList" value="{{item.ProductId}}">{{item.Name}}</option>
</select>
</div>
<div class="form-group col-md-6">
<select class="form-control" ng-model="ProductStock.BatchId">
<option ng-repeat="item in BatchList" value="{{item.BatchId}}">{{item.BatchName}}</option>
</select>
</div>
<div class="form-group col-md-6">
<input type="number" class="form-control form-control-user" ng-model="ProductStock.Quantity" placeholder="Quantity">
</div>
<div class="form-group col-md-6">
<input type="number" class="form-control form-control-user" ng-model="ProductStock.PurchasePrice" placeholder="Purchase Price">
</div>
<div class="form-group col-md-6">
<input type="number" class="form-control form-control-user" ng-model="ProductStock.SalesPrice" placeholder="Sales Price">
</div>
<div class="form-group col-md-6">
<a href="#" ng-click="SaveProductStock()" class="btn btn-primary btn-sm">
Save
</a>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
<br />
<div class="col-xl-12 col-md-12 mb-12">
<div class="card border-left-primary shadow h-100 py-2">
<div class="card-body">
<div class="row align-items-center">
<div class="col mr-2">
<div class="form-group col-md-6">
<div class="text-xs font-weight-bold text-primary text-uppercase mb-1">Stock List</div>
</div>
<table class="table table-bordered" width="100%" cellspacing="0">
<thead>
<tr>
<th>Product Name</th>
<th>Batch name</th>
<th>Quantity</th>
<th>Purchase Price</th>
<th>Sales Price</th>
<th>
Action
</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="item in ProductStockList">
<td>{{item.ProductName}}</td>
<td>{{item.BatchName}}</td>
<td>{{item.Quantity}}</td>
<td>{{item.PurchasePrice}}</td>
<td>{{item.SalesPrice}}</td>
<td>Edit</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
</div>
<script src="~/Scripts/Home/HomeProductStockController.js"></script>
Step-2
The main part of this ProductStock CRUD is saving, updating, retrieving data so here I create and update data using one single method SaveCategory
- Please go to HomeController
- Add this below method inside the HomeController
[HttpPost]
public JsonResult SaveProductStock(ProductStock stock)
{
PointOfSale.Helper.AppHelper.ReturnMessage retMessage = new AppHelper.ReturnMessage();
POS_TutorialEntities db = new POS_TutorialEntities();
retMessage.IsSuccess = true;
if (stock.ProductQtyId > 0)
{
db.Entry(stock).State = EntityState.Modified;
retMessage.Messagae = "Update Success!";
}
else
{
db.ProductStocks.Add(stock);
retMessage.Messagae = "Save Success!";
}
try
{
db.SaveChanges();
}
catch (Exception)
{
retMessage.IsSuccess = false;
}
return Json(retMessage, JsonRequestBehavior.AllowGet);
}
Step-3
As a part of Stock Insert data we must show list of data to select for updating. Please follow below steps to
- Please go to HomeController.
- Please write this below code to show list of Product Stock.
[HttpGet]
public JsonResult GetAllProductStocks()
{
POS_TutorialEntities db = new POS_TutorialEntities();
var dataList = db.ProductStocks.Include("Product").Include("Batch").ToList();
var modefiedData = dataList.Select(x => new
{
ProductQtyId = x.ProductQtyId,
ProductId = x.ProductId,
ProductName=x.Product.Name,
Quantity=x.Quantity,
BatchId=x.BatchId,
BatchName=x.Batch.BatchName,
PurchasePrice=x.PurchasePrice,
SalesPrice=x.SalesPrice
}).ToList();
return Json(modefiedData, JsonRequestBehavior.AllowGet);
}
Step-4
As we are implementing CRUD operation here we used Here AngularJS JSON, AJAX so we will add A JavaScript file which will save, update, retrieve data.
- Create a folder under script called Home
- Please go to Script>Home then create a JS file called HomeProductStockController.js
- Please add this below code in HomeProductStockController.js.
(function () {
app.controller('ProductStock', function ($scope, $http) {
$scope.ProductStock = new Object();
var init = function () {
GetProducts();
GetBatchs();
GetAllProductStock();
}; //end of init
init(); //init is called
function GetProducts() {
$.ajax({
contentType: 'application/json; charset=utf-8',
dataType: 'json',
type: 'Get',
url: "/Home/GetAllProduct",
success: function (data) {
$scope.ProductList = data;
},
error: function () {
alert("Error!")
}
});
}
function GetBatchs() {
$.ajax({
contentType: 'application/json; charset=utf-8',
dataType: 'json',
type: 'Get',
url: "/Home/GetAllBatch",
success: function (data) {
$scope.BatchList = data;
},
error: function () {
alert("Error!")
}
});
}
$scope.SaveProductStock = function () {
var data = JSON.stringify({
stock: $scope.ProductStock
});
return $.ajax({
contentType: 'application/json; charset=utf-8',
dataType: 'json',
type: 'POST',
url: "/Home/SaveProductStock",
data: data,
success: function (result) {
if (result.IsSuccess == true) {
//GetAllProduct();
//Reset();
alert("Save Success!");
}
else {
alert("Save failed!");
}
},
error: function () {
alert("Error!")
}
});
}
function GetAllProductStock() {
$.ajax({
contentType: 'application/json; charset=utf-8',
dataType: 'json',
type: 'Get',
url: "/Home/GetAllProductStocks",
success: function (data) {
$scope.ProductStockList = data;
},
error: function () {
alert("Error!")
}
});
}
});
}).call(angular);
- Now run the project.
- Then save, update, retrieve data.