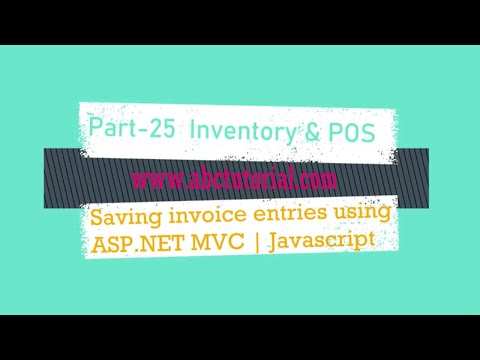
Part-1: Point of Sale(POS) Inventory Super Shop Management System using ASP.NET MVC
Part-2: Point of Sale(POS) Setup template to your project | Super Shop Management System|ASP.NET MVC
Part-3: Point of Sale(POS) Database & table analysis | Super Shop Management System|ASP.NET MVC
Part-4: Point of Sale(POS) Database Create in SQL Server | Super Shop Management System|ASP.NET MVC
Part-5: Point of Sale(POS) Login using AJAX ASP.NET MVC | JQUERY
Part-6: Point of Sale(POS) Login and Authorization with Session variables in ASP.NET | JQUERY | AJAX
Part-7: Point of Sale(POS) Convert Password to MD5 Hash string in ASP.NET | Encrypt password in ASP.NET MVC C#
Part-8: Point of Sale(POS) Role based authentication and authorization in ASP.NET MVC|Redirect to not found page if not authorized|C#
Part-9: Point of Sale(POS) Create user & Account management UI UX in ASP.NET MVC
Part-10: Point of Sale(POS) User Creation & user registration using ASP.NET MVC | Jquery | Ajax
Part-11: Point of Sale(POS) Get user list using ASP.NET MVC | Jquery | Ajax
Part-12: Point of Sale(POS) Update user using ASP.NET MVC | Jquery | Ajax
Part-13: Inventory and POS Login logout session using ASP.NET | Supershop management system
Part-14: Inventory Category CRUD Create,Retrieve,Update,View | POS Category CRUD using ASP.NET JQuery AJAX
Part-15: Inventory and POS batch tracking and control table create in SQL Server 2012
Part-16: Inventory Product CRUD List | Point of sale Products Crud using asp.net MVC
Part-17: Inventory and POS Batch CRUD using asp.net MVC JQUERY AJAX | CSharp
Part-18: Inventory management and stock control using asp.net mvc | Jquery AngularJs
Part-19: Inventory & POS Stock edit and validation using asp.net AngularJS
POS 20: Inventory & POS AngularJS error fix
POS-21: Inventory & POS Invoice template setup using Bootstrap
POS-22: Invoice Adding input fields & adding rows Dynamically using Javascript | ASP.NET MVC | AngularJs
POS-23: Inventory Onclick get selected data & Calculate line total using Javascript ASP.NET MVC JQUERY
POS-24: Inventory Invoice Configuration and Calculation using JavaScript AngularJS ASP.NET MVC
POS-25: Inventory sale from invoice using ASP.NET MVC | Jquery AngularJS
POS-26: Get data using ASP.NET MVC AngularJS JQUERY
POS-27: Invoice sales page edit using ASP.NET MVC JQUERY AngularJS
POS-28: Invoice vat calculation discount calculation using Javascript ASP.NET MVC | Invoice crud asp.net
POS-29: Invoice calculate subtotal add row remove row using AngularJS ASP.NET MVC
Post your any code related problem to www.abctutorial.com
Follow the previous video and articles to complete the POS tutorials
Step-1:
We will show Order number as auto generated guid Id.
- Use this code to show Guid id in the Order page
ViewBag.InvoiceNum = Guid.NewGuid();
- Put this code to show the Order number in Invoice page.
<strong>@ViewBag.InvoiceNum</strong>
- Use this input box to carry Order number as hidden to save.
<input type="hidden" id="OrderNo" value="@ViewBag.InvoiceNum"/>
- Use this code to add ng-model to Customer name,address,phone
<div>
<input type="text" ng-model="Sale.CustomerName" class="BorderLess" placeholder="Customer name" />
</div>
<div><textarea class="BorderLess" ng-model="Sale.CustomerAddress" placeholder="Address"></textarea></div>
<div><input class="BorderLess" ng-model="Sale.CustomerPhone" type="number" placeholder="Phone" /></div>
Step-2:
- Add SaveInvoiceSale method inside your HomeController.cs
[HttpPost]
public JsonResult SaveInvoiceSale(Sale sale, List<SalesDetail> salesDetails)
{
PointOfSale.Helper.AppHelper.ReturnMessage retMessage = new AppHelper.ReturnMessage();
POS_TutorialEntities db = new POS_TutorialEntities();
retMessage.IsSuccess = true;
foreach (var item in salesDetails)
{
sale.SalesDetails.Add(new SalesDetail { ProductId = item.ProductId, UnitPrice = item.UnitPrice, Quantity = item.Quantity, LineTotal = item.LineTotal });
var prd = db.ProductStocks.Where(x => x.ProductId == item.ProductId && x.Quantity > 0).FirstOrDefault();
prd.Quantity = prd.Quantity - item.Quantity;
db.Entry(prd).State = EntityState.Modified;
}
db.Sales.Add(sale);
retMessage.Messagae = "Save Success!";
try
{
db.SaveChanges();
}
catch (Exception)
{
retMessage.IsSuccess = false;
}
return Json(retMessage, JsonRequestBehavior.AllowGet);
}
- Add SaveInvoice method inside the HomeInvoiceController.js to save data.
$scope.SaveInvoice = function () {
$scope.Sale.OrderNo = $("#OrderNo").val();
var data = JSON.stringify({
sale: $scope.Sale, salesDetails: $scope.InvoiceCart
});
return $.ajax({
contentType: 'application/json; charset=utf-8',
dataType: 'json',
type: 'POST',
url: "/Home/SaveInvoiceSale",
data: data,
success: function (result) {
if (result.IsSuccess == true) {
//Reset();
alert("Save Success!");
}
else {
alert("Save failed!");
}
},
error: function () {
alert("Error!")
}
});
}
- Add a button to click and save. Use SaveInvoice method name inside the ng-click.
<a class="btn btn-success" ng-click="SaveInvoice()">Save</a>
- Run the project and see the output